"driver_path": "C:\Program Files\Google\Chrome\Application",
时间: 2023-07-28 09:11:23 浏览: 170
在你提供的示例中,`"driver_path": "C:\Program Files\Google\Chrome\Application"` 这个字符串中的反斜杠 (`\`) 被认为是一个无效的转义字符。这是因为在 JSON 中,反斜杠需要进行转义,即用两个反斜杠 (`\\`) 来表示一个反斜杠。
要修复这个问题,你可以将字符串中的反斜杠转义为双反斜杠 (`\\`),像这样:
```json
"driver_path": "C:\\Program Files\\Google\\Chrome\\Application"
```
这样就能确保 JSON 解析器正确地识别和处理转义字符。
相关问题
from selenium import webdriver # 设置Chrome浏览器驱动程序的路径 driver_path = "C:\Program Files\Google\Chrome\Application/chromedriver" # 创建Chrome浏览器对象 driver = webdriver.Chrome(driver_path) # 打开百度网页 driver.get("https://www.baidu.com") # 找到搜索框元素,并输入关键字 search_box = driver.find_element_by_css_selector("#kw") search_box.send_keys("RPA") # 找到搜索按钮元素,并点击 search_button = driver.find_element_by_css_selector("#su") search_button.click() # 等待搜索结果加载完成 driver.implicitly_wait(5) # 获取搜索结果列表 search_results = driver.find_elements_by_css_selector(".result") # 打印搜索结果标题和链接 for result in search_results: title = result.find_element_by_css_selector("h3").text url = result.find_element_by_css_selector("a").get_attribute("href") print(f"Title: {title}") print(f"URL: {url}") print("") # 关闭浏览器 driver.quit() 将读取的数据保存成pdf
要将搜索结果保存为PDF文件,你可以使用第三方库来实现,例如使用`pdfkit`库。
首先,你需要安装`pdfkit`库。在命令行中运行以下命令:
```
pip install pdfkit
```
然后,你需要下载并安装wkhtmltopdf工具,它是将HTML转换为PDF的工具。下载地址:https://wkhtmltopdf.org/downloads.html
安装完成后,你可以按照以下方式修改你的代码来保存搜索结果为PDF文件:
```python
import pdfkit
from selenium import webdriver
# 设置Chrome浏览器驱动程序的路径
driver_path = "C:\Program Files\Google\Chrome\Application/chromedriver"
# 创建Chrome浏览器对象
driver = webdriver.Chrome(driver_path)
# 打开百度网页
driver.get("https://www.baidu.com")
# 找到搜索框元素,并输入关键字
search_box = driver.find_element_by_css_selector("#kw")
search_box.send_keys("RPA")
# 找到搜索按钮元素,并点击
search_button = driver.find_element_by_css_selector("#su")
search_button.click()
# 等待搜索结果加载完成
driver.implicitly_wait(5)
# 获取搜索结果列表的HTML内容
search_results_html = driver.find_element_by_css_selector("body").get_attribute("innerHTML")
# 关闭浏览器
driver.quit()
# 将HTML内容保存为PDF文件
pdfkit.from_string(search_results_html, "search_results.pdf")
```
运行以上代码后,它将会将搜索结果保存为名为`search_results.pdf`的PDF文件。请确保`pdfkit`和`wkhtmltopdf`都已正确安装,并且`wkhtmltopdf`的路径已添加到系统环境变量中。
import os import time import platform from selenium import webdriver as webdriver1 from selenium.webdriver.ie.options import Options from selenium.webdriver.support import expected_conditions as EC from selenium.webdriver.support.ui import WebDriverWait from selenium.webdriver.common.by import By from config import global_wait,root_url,use_edge,iedriver_path,edgedriver_path,chromedriver_path from public.basiclogger import LOGGING class BaseCase(object): '''基础用例''' def get_web_driver(self,url,data=None,browser='ie'): if browser=='chrome': #if platform.system()=="Windows": # os.system(r'taskkill /f /im chrome.exe') #else: # os.system(r"ps aux |grep chrome|awk '{print $2}'|xargs -i kill {}") options = webdriver1.ChromeOptions() options.add_experimental_option('excludeSwitches', ['enable-logging']) self.driver = webdriver1.Chrome(executable_path=chromedriver_path,options=options) self.driver.get(url) self.driver.maximize_window() self.driver.implicitly_wait(global_wait) else: #启动IE之前先杀死电脑上所有的IE if use_edge: os.system(r'taskkill /f /im msedge.exe') else: os.system(r'taskkill /f /im iexplore.exe') if use_edge: file1=edgedriver_path else: file1=iedriver_path options = Options() options.ignore_protected_mode_settings = True options.ignore_zoom_level = True if use_edge: options.attach_to_edge_chrome = True options.edge_executable_path = r"C:\Program Files (x86)\Microsoft\Edge\Application\msedge.exe" else: options.add_argument('--user-agent="Mozilla/5.0 (Windows NT 10.0; WOW64; Trident/7.0; rv:11.0) like Gecko"') self.driver=webdriver1.Ie(executable_path=file1,options=options) self.driver.get(url) self.driver.maximize_window() self.driver.implicitly_wait(global_wait) def get_logger(self): #创建日志文件名 filename=self.data["case_name"]+'_'+time.strftime("%Y-%m-%d_%H-%M-%S") #保存连接 filename1=os.path.join(r'test_log',filename+r'.log') LOGGING1=LOGGING(log_name=filename,log_dir="test_log") self.logger=LOGGING1.logObject self.LOGGER=LOGGING1
在您提供的代码中,第一行需要将多个导入语句分开,即:
```
import os
import time
import platform
from selenium import webdriver as webdriver1
from selenium.webdriver.ie.options import Options
from selenium.webdriver.support import expected_conditions as EC
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.common.by import By
from config import global_wait,root_url,use_edge,iedriver_path,edgedriver_path,chromedriver_path
from public.basiclogger import LOGGING
```
此外,我还注意到您的代码缩进存在问题,建议仔细检查缩进是否正确,以确保代码可以正确执行。
阅读全文
相关推荐
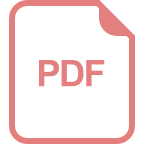
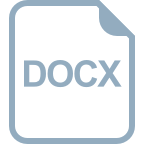
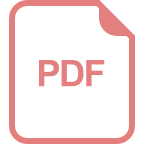














