调用百度长视频审核API()使用java语言,提交视频审核任务,接收视频审核结果,拉取视频审核写一段示例代码
时间: 2024-06-09 22:06:02 浏览: 156
以下是使用Java语言调用百度长视频审核API的示例代码:
```java
import com.alibaba.fastjson.JSON;
import com.alibaba.fastjson.JSONArray;
import com.alibaba.fastjson.JSONObject;
import org.apache.commons.codec.binary.Base64;
import org.apache.http.HttpEntity;
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.ContentType;
import org.apache.http.entity.mime.MultipartEntityBuilder;
import org.apache.http.entity.mime.content.FileBody;
import org.apache.http.entity.mime.content.StringBody;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClientBuilder;
import org.apache.http.util.EntityUtils;
import java.io.File;
import java.io.IOException;
public class VideoAuditExample {
private static final String API_URL = "https://aip.baidubce.com/rest/2.0/solution/v1/video_censor/v2/user_defined";
private static final String API_KEY = "your_api_key";
private static final String SECRET_KEY = "your_secret_key";
public static void main(String[] args) throws IOException {
String filePath = "/path/to/your/video/file.mp4";
String result = submitVideoAuditTask(filePath);
System.out.println(result);
}
private static String submitVideoAuditTask(String filePath) throws IOException {
CloseableHttpClient httpClient = HttpClientBuilder.create().build();
HttpPost httpPost = new HttpPost(API_URL);
String accessToken = getAccessToken(API_KEY, SECRET_KEY);
httpPost.setHeader("Content-Type", "multipart/form-data");
httpPost.setHeader("Authorization", "Bearer " + accessToken);
FileBody fileBody = new FileBody(new File(filePath));
StringBody parameters = new StringBody("{\"scenes\": [\"antiporn\", \"terror\"], \"antiporn\": {\"interval\": 5}}, ContentType.APPLICATION_JSON");
HttpEntity entity = MultipartEntityBuilder.create()
.addPart("video", fileBody)
.addPart("params", parameters)
.build();
httpPost.setEntity(entity);
CloseableHttpResponse response = httpClient.execute(httpPost);
HttpEntity responseEntity = response.getEntity();
String result = EntityUtils.toString(responseEntity);
JSONObject jsonObject = JSON.parseObject(result);
String taskId = jsonObject.getString("taskId");
// 拉取视频审核结果
result = getVideoAuditResult(taskId, accessToken);
return result;
}
private static String getVideoAuditResult(String taskId, String accessToken) throws IOException {
String url = "https://aip.baidubce.com/rest/2.0/solution/v1/video_censor/v2/user_defined/result?taskId=" + taskId;
CloseableHttpClient httpClient = HttpClientBuilder.create().build();
HttpPost httpPost = new HttpPost(url);
httpPost.setHeader("Content-Type", "application/json");
httpPost.setHeader("Authorization", "Bearer " + accessToken);
CloseableHttpResponse response = httpClient.execute(httpPost);
HttpEntity responseEntity = response.getEntity();
String result = EntityUtils.toString(responseEntity);
JSONArray jsonArray = JSON.parseArray(result);
JSONObject jsonObject = jsonArray.getJSONObject(0);
String status = jsonObject.getString("status");
if ("SUCCESS".equals(status)) {
return result;
} else {
return "审核失败";
}
}
private static String getAccessToken(String apiKey, String secretKey) throws IOException {
CloseableHttpClient httpClient = HttpClientBuilder.create().build();
HttpPost httpPost = new HttpPost("https://aip.baidubce.com/oauth/2.0/token");
httpPost.setHeader("Content-Type", "application/x-www-form-urlencoded");
String authString = apiKey + ":" + secretKey;
byte[] authBytes = authString.getBytes();
String encodedAuthString = new String(Base64.encodeBase64(authBytes));
StringBody parameters = new StringBody("grant_type=client_credentials&client_id=" + apiKey + "&client_secret=" + secretKey, ContentType.APPLICATION_FORM_URLENCODED);
HttpEntity entity = MultipartEntityBuilder.create()
.addPart("grant_type=client_credentials&client_id=" + apiKey + "&client_secret=" + secretKey, parameters)
.build();
httpPost.setEntity(entity);
CloseableHttpResponse response = httpClient.execute(httpPost);
HttpEntity responseEntity = response.getEntity();
String result = EntityUtils.toString(responseEntity);
JSONObject jsonObject = JSON.parseObject(result);
String accessToken = jsonObject.getString("access_token");
return accessToken;
}
}
```
其中,`API_KEY`和`SECRET_KEY`需要替换成你的百度API Key和Secret Key。`filePath`需要替换成你要审核的视频文件路径。该示例代码提交视频审核任务后,会返回任务ID,然后通过任务ID去拉取视频审核结果。
阅读全文
相关推荐
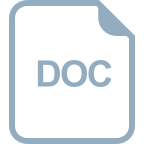
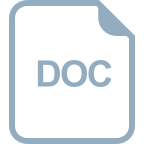
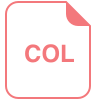
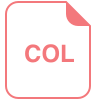
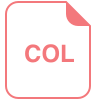
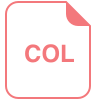
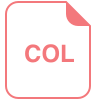
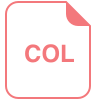
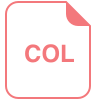
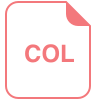
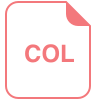
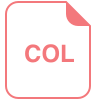
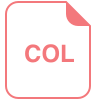
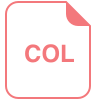
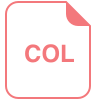