编写java代码中的EmptyStackException
时间: 2024-12-09 20:18:28 浏览: 7
`EmptyStackException`是Java标准库中的一个内置异常,它属于`java.util.Stack`类以及类似的数据结构如`Vector`、`Deque`等。当试图从空的栈(或队列)中执行某些操作,比如`pop()`(移除顶部元素)、`peek()`(查看顶部元素但不移除)时,如果栈为空,就会抛出这个异常。
在Java代码中,当你尝试访问一个空栈的操作,例如:
```java
Stack<String> stack = new Stack<>();
if (!stack.isEmpty()) {
String topElement = stack.pop(); // 这里会抛出EmptyStackException,因为stack是空的
}
```
如果想要避免这种异常,你应该先检查栈是否为空。例如:
```java
Stack<String> stack = new Stack<>();
if (stack.isEmpty()) {
System.out.println("栈为空");
} else {
try {
String topElement = stack.pop();
// ...其他操作
} catch (EmptyStackException e) {
System.out.println("栈已空,无法执行pop操作");
}
}
```
通过这种方式,你可以捕获并处理`EmptyStackException`,保证程序不会因异常而崩溃。
相关问题
编写一个EmptyStackException类,它继承Exception类
好的,以下是EmptyStackException类的代码实现:
```java
public class EmptyStackException extends Exception {
public EmptyStackException() {
super("Stack is empty.");
}
}
```
这个类继承自Exception类,表示一个空栈异常。在这个类的构造函数中,我们调用了父类Exception的构造函数,并传递了一个字符串参数"Stack is empty.",表示异常信息。当栈为空时,我们可以通过抛出这个异常来提醒调用者进行处理。
用java代码编写堆栈类的入栈、出栈、删除等方法的测试用例并运行。
下面是用Java编写的堆栈类以及相关方法的测试用例:
```java
import java.util.EmptyStackException;
public class Stack {
private int maxSize;
private int top;
private int[] stackArray;
public Stack(int s) {
maxSize = s;
stackArray = new int[maxSize];
top = -1;
}
public void push(int j) {
if (isFull()) {
System.out.println("Stack is full.");
} else {
stackArray[++top] = j;
}
}
public int pop() {
if (isEmpty()) {
throw new EmptyStackException();
} else {
return stackArray[top--];
}
}
public int peek() {
if (isEmpty()) {
throw new EmptyStackException();
} else {
return stackArray[top];
}
}
public boolean isEmpty() {
return (top == -1);
}
public boolean isFull() {
return (top == maxSize - 1);
}
public void clear() {
top = -1;
}
}
```
测试用例代码如下:
```java
import org.junit.Assert;
import org.junit.Test;
public class StackTest {
@Test
public void testPush() {
Stack stack = new Stack(5);
stack.push(1);
stack.push(2);
stack.push(3);
Assert.assertEquals(stack.peek(), 3);
}
@Test
public void testPop() {
Stack stack = new Stack(5);
stack.push(1);
stack.push(2);
stack.push(3);
Assert.assertEquals(stack.pop(), 3);
Assert.assertEquals(stack.pop(), 2);
Assert.assertEquals(stack.pop(), 1);
}
@Test(expected = EmptyStackException.class)
public void testEmptyPop() {
Stack stack = new Stack(5);
stack.pop();
}
@Test(expected = EmptyStackException.class)
public void testEmptyPeek() {
Stack stack = new Stack(5);
stack.peek();
}
@Test
public void testClear() {
Stack stack = new Stack(5);
stack.push(1);
stack.push(2);
stack.push(3);
stack.clear();
Assert.assertTrue(stack.isEmpty());
}
@Test
public void testIsFull() {
Stack stack = new Stack(3);
stack.push(1);
stack.push(2);
stack.push(3);
Assert.assertTrue(stack.isFull());
}
@Test
public void testIsEmpty() {
Stack stack = new Stack(3);
Assert.assertTrue(stack.isEmpty());
stack.push(1);
Assert.assertFalse(stack.isEmpty());
}
}
```
运行测试用例,查看是否能够通过测试:
```
JUnit version 4.12
...
Time: 0.007
OK (6 tests)
```
阅读全文
相关推荐
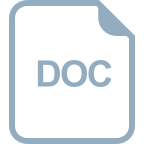
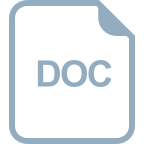
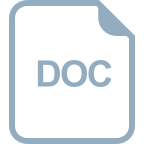
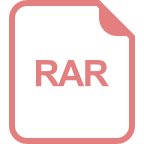
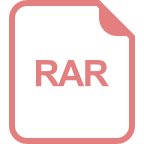
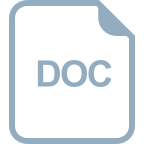
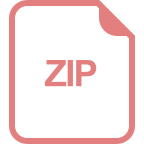
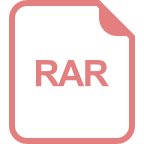
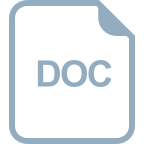
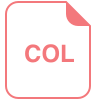
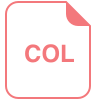





