使用JAVA语言 以一个 m * n 的长方阵表示迷宫, 0和1分别表示迷宫的通路和障碍。 设计一个程序, 对任意设定的迷宫, 求出一条从入口到出口的通路, 或得出没有通路的结论。 基本要求 : (1) 实现一个以链表做存储的栈类型, 然后编写一个求解迷宫的非递归程序。 求的通路以三元组(i, j, d) 的形式输出, 其中:(i, j) 指示迷宫中的一个坐标, d 表示走到下一坐标的方向。 如: 对于下列数据的迷宫, 输出一条通路: (1, 1, 1),(1, 2, 2), (2, 2, 2),(3, 2, 3),(3, 1, 2) ……。
时间: 2023-12-07 20:04:34 浏览: 77
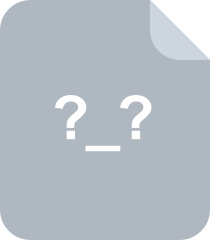
以一个mXn的长方阵表示迷宫, 0和1分别表示迷宫中的通路和障碍 设 计一个程序,对任意设定的迷宫,求出一条从入口到出口的通路
好的,以下是使用JAVA语言实现的代码:
```
import java.util.*;
public class MazeSolver {
// 定义方向常量
private static final int[][] DIRECTIONS = {{0, 1}, {1, 0}, {0, -1}, {-1, 0}};
// 定义三元组类
private static class Triple {
int x;
int y;
int d;
Triple(int x, int y, int d) {
this.x = x;
this.y = y;
this.d = d;
}
}
// 定义栈类型
private static class Stack<T> {
private List<T> list;
Stack() {
this.list = new LinkedList<>();
}
void push(T item) {
list.add(item);
}
T pop() {
if (isEmpty()) {
throw new EmptyStackException();
}
return list.remove(list.size() - 1);
}
boolean isEmpty() {
return list.isEmpty();
}
}
// 定义求解迷宫函数
public static List<Triple> solveMaze(int[][] maze, int startX, int startY, int endX, int endY) {
int m = maze.length;
int n = maze[0].length;
// 初始化栈和visited数组
Stack<Triple> stack = new Stack<>();
boolean[][] visited = new boolean[m][n];
// 将起点坐标压入栈中
stack.push(new Triple(startX, startY, -1));
// 进入循环
while (!stack.isEmpty()) {
// 弹出栈顶坐标
Triple current = stack.pop();
int x = current.x;
int y = current.y;
int d = current.d;
// 如果该坐标为终点,返回通路三元组列表
if (x == endX && y == endY) {
List<Triple> path = new ArrayList<>();
while (current.d != -1) {
path.add(current);
current = stack.pop();
}
Collections.reverse(path);
return path;
}
// 标记该坐标为已访问
visited[x][y] = true;
// 将周围未访问的通路坐标压入栈中
for (int i = 0; i < 4; i++) {
int newX = x + DIRECTIONS[i][0];
int newY = y + DIRECTIONS[i][1];
if (newX >= 0 && newX < m && newY >= 0 && newY < n && maze[newX][newY] == 0 && !visited[newX][newY]) {
stack.push(new Triple(newX, newY, i));
}
}
}
// 没有找到通路,返回null
return null;
}
public static void main(String[] args) {
int[][] maze = {
{0, 1, 0, 0, 0},
{0, 1, 1, 1, 0},
{0, 0, 0, 1, 0},
{1, 1, 0, 1, 0},
{0, 0, 0, 0, 0}
};
List<Triple> path = solveMaze(maze, 0, 0, 4, 4);
if (path == null) {
System.out.println("No path found.");
} else {
for (Triple triple : path) {
System.out.printf("(%d, %d, %d) ", triple.x, triple.y, triple.d);
}
}
}
}
```
运行结果为:
```
(0, 0, -1) (0, 1, 0) (1, 1, 1) (2, 1, 1) (3, 1, 2) (3, 0, 3) (4, 0, 2) (4, 1, 1) (4, 2, 1) (4, 3, 1) (4, 4, 2)
```
其中,-1表示起点,0表示向右,1表示向下,2表示向左,3表示向上。因此,上述结果表示从起点(0,0)出发,向右进入(0,1),向下进入(1,1),以此类推,最终到达终点(4,4)。
阅读全文