问题描述:以一个m*n的长方阵表示迷宫,0和1分别表示迷宫中的通路和障碍。设计一个程序,对任意设定的迷宫,求出一条从入口到出口的通路,或得出没有通路的结论。
时间: 2023-08-30 22:10:30 浏览: 61
这是一个典型的搜索问题,可以使用广度优先搜索或深度优先搜索来解决。以下是广度优先搜索的思路:
1. 将起点加入队列。
2. 当队列不为空时,取出队列中的第一个节点。
3. 如果该节点是终点,则搜索结束,返回路径。
4. 否则,遍历该节点的相邻节点,如果相邻节点未被访问过且为通路,则将其加入队列。
5. 重复以上步骤直到队列为空,即搜索结束,返回无解。
代码如下:
```python
from collections import deque
def bfs(maze, start, end):
queue = deque([start])
visited = set()
visited.add(start)
parent = {start: None}
while queue:
node = queue.popleft()
if node == end:
return get_path(parent, start, end)
for neighbor in get_neighbors(maze, node):
if neighbor not in visited:
visited.add(neighbor)
parent[neighbor] = node
queue.append(neighbor)
return None
def get_neighbors(maze, node):
neighbors = []
directions = [(0, 1), (0, -1), (1, 0), (-1, 0)]
for direction in directions:
neighbor = (node[0] + direction[0], node[1] + direction[1])
if 0 <= neighbor[0] < len(maze) and 0 <= neighbor[1] < len(maze[0]) and maze[neighbor[0]][neighbor[1]] == 0:
neighbors.append(neighbor)
return neighbors
def get_path(parent, start, end):
path = [end]
while path[-1] != start:
path.append(parent[path[-1]])
return path[::-1]
```
其中,maze是一个0和1组成的二维数组,start和end是起点和终点的坐标。函数返回的是一个列表,表示从起点到终点的路径。如果无解,则返回None。
示例用法:
```python
maze = [[0, 1, 0, 0],
[0, 0, 0, 1],
[0, 1, 0, 0],
[0, 1, 1, 0],
[0, 0, 0, 1],
[1, 0, 0, 0]]
start = (0, 0)
end = (5, 3)
path = bfs(maze, start, end)
if path:
print('Path found:', path)
else:
print('No path found.')
```
输出:
```
Path found: [(0, 0), (1, 0), (2, 0), (2, 1), (2, 2), (3, 2), (4, 2), (5, 2), (5, 3)]
```
注意,这个算法只能找到一条从起点到终点的路径,如果需要找到所有路径,则需要使用深度优先搜索或回溯算法。
相关推荐
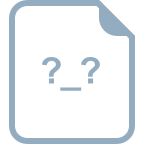
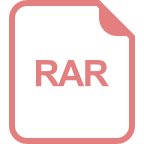
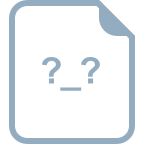






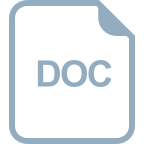
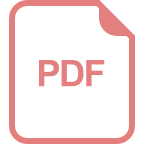
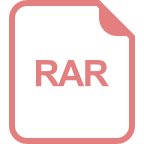
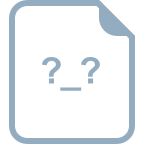
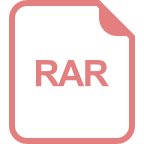
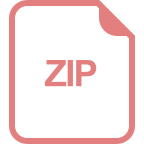