以一个m*n的长方阵表示迷宫,0和1分别表示迷宫中的通路和障碍。 设计一个程序,对任意设定的迷宫,求出一条从入口到出口的最短通路,或得出没有通路的结论
时间: 2024-09-11 22:02:02 浏览: 121
要解决这个问题,我们可以使用广度优先搜索(BFS)算法来寻找从入口到出口的最短路径。BFS是一种遍历或搜索树或图的算法,它从根节点开始,逐层向外扩展,直到找到所需的节点。在迷宫中,我们将从入口开始,探索所有可能的通路,直到找到出口。
以下是该算法的基本步骤:
1. 创建一个与迷宫大小相同的队列,用于存放待探索的节点。
2. 创建一个同样大小的矩阵,用于标记节点的访问状态和记录路径。
3. 将入口节点加入队列,并将其在访问矩阵中标记为已访问。
4. 如果队列非空,循环执行以下操作:
a. 从队列中取出一个节点,检查该节点是否为出口。如果是,记录路径并结束搜索。
b. 将该节点四周的通路节点(值为0且未被访问过)加入队列,并在访问矩阵中标记为已访问。
c. 为每个加入队列的节点记录其父节点,以便最后可以追溯出一条路径。
5. 如果所有通路节点都被访问过,而队列为空,则说明没有通路可以到达出口。
伪代码示例如下:
```pseudo
function BFS(maze, start, end):
rows, cols = maze.length, maze[0].length
visited = matrix of size rows * cols, initialized with false
queue = queue of node pairs (x, y)
parent = matrix of size rows * cols, initialized with null
if maze[start.x][start.y] == 0:
queue.enqueue((start.x, start.y))
visited[start.x][start.y] = true
parent[start.x][start.y] = null
directions = [(0, 1), (1, 0), (0, -1), (-1, 0)] // 右、下、左、上
while not queue.isEmpty():
(x, y) = queue.dequeue()
if (x, y) == end:
return reconstructPath(parent, start, end)
for (dx, dy) in directions:
newX, newY = x + dx, y + dy
if newX >= 0 and newY >= 0 and newX < rows and newY < cols and
maze[newX][newY] == 0 and not visited[newX][newY]:
queue.enqueue((newX, newY))
visited[newX][newY] = true
parent[newX][newY] = (x, y)
return null // 没有找到通路
function reconstructPath(parent, start, end):
path = []
while end is not null:
path.prepend(end)
end = parent[end.x][end.y]
return path
```
注意:`reconstructPath`函数用于回溯找到的路径,从出口开始,按照每个节点的父节点向入口方向追溯,直到到达起点。
阅读全文
相关推荐
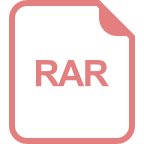






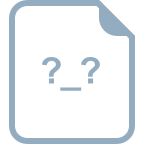
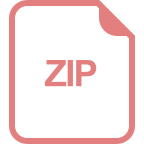
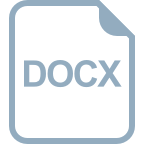