bfs and dfs java code
时间: 2023-08-31 15:09:54 浏览: 47
Here is the Java code for BFS and DFS:
BFS:
```
import java.util.*;
public class BFS {
public void bfs(int[][] adjMatrix, int start) {
Queue<Integer> queue = new LinkedList<>();
boolean[] visited = new boolean[adjMatrix.length];
visited[start] = true;
queue.offer(start);
while (!queue.isEmpty()) {
int current = queue.poll();
System.out.print(current + " ");
for (int i = 0; i < adjMatrix.length; i++) {
if (adjMatrix[current][i] == 1 && !visited[i]) {
visited[i] = true;
queue.offer(i);
}
}
}
}
}
```
DFS:
```
import java.util.*;
public class DFS {
public void dfs(int[][] adjMatrix, int start) {
Stack<Integer> stack = new Stack<>();
boolean[] visited = new boolean[adjMatrix.length];
visited[start] = true;
stack.push(start);
while (!stack.isEmpty()) {
int current = stack.pop();
System.out.print(current + " ");
for (int i = 0; i < adjMatrix.length; i++) {
if (adjMatrix[current][i] == 1 && !visited[i]) {
visited[i] = true;
stack.push(i);
}
}
}
}
}
```
相关推荐
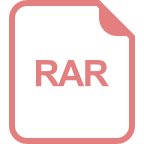
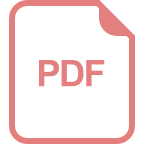
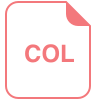













