用c++设计一个用于人事管理的人员类。这个类要包含人员的编号,姓名,性别,出生日期,身份证号等。其中出生日期定义为一个日期类内嵌紫对象
时间: 2023-09-15 13:24:16 浏览: 97
好的,下面是一个简单的人员类设计:
```cpp
#include <string>
#include <iostream>
class Date {
public:
Date(int year, int month, int day) : year_(year), month_(month), day_(day) {}
friend std::ostream& operator<<(std::ostream& os, const Date& date) {
os << date.year_ << "/" << date.month_ << "/" << date.day_;
return os;
}
private:
int year_;
int month_;
int day_;
};
class Person {
public:
Person(int id, const std::string& name, char gender, const Date& birthday, const std::string& id_num)
: id_(id), name_(name), gender_(gender), birthday_(birthday), id_num_(id_num) {}
friend std::ostream& operator<<(std::ostream& os, const Person& person) {
os << "ID: " << person.id_ << std::endl
<< "Name: " << person.name_ << std::endl
<< "Gender: " << person.gender_ << std::endl
<< "Birthday: " << person.birthday_ << std::endl
<< "ID Number: " << person.id_num_ << std::endl;
return os;
}
private:
int id_;
std::string name_;
char gender_;
Date birthday_;
std::string id_num_;
};
```
在这个类中,我们使用了内嵌日期类 `Date` 来定义出生日期。`Person` 类中包含了人员编号、姓名、性别、出生日期和身份证号等信息,并重载了流输出运算符,方便输出人员信息。
下面是使用示例:
```cpp
int main() {
Date birthday(1990, 10, 1);
Person person(1, "张三", 'M', birthday, "123456789012345678");
std::cout << person;
return 0;
}
```
输出结果为:
```
ID: 1
Name: 张三
Gender: M
Birthday: 1990/10/1
ID Number: 123456789012345678
```
相关推荐
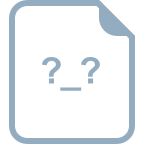
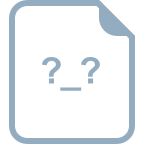














