在python中,在词频统计的时候不要统计标点符号
时间: 2024-03-15 11:47:34 浏览: 70
当然可以,在Python中,你可以使用正则表达式来匹配并删除标点符号,然后再进行词频统计。以下是一个简单的示例代码:
```python
import re
from collections import Counter
text = "Hello, world! This is a sample text. It contains punctuation marks and we need to remove them."
# 使用正则表达式删除标点符号
text = re.sub(r'[^\w\s]', '', text)
# 将文本分割成单词,并计算词频
word_freq = Counter(text.lower().split())
# 打印结果
print(word_freq)
```
输出结果如下:
```
Counter({'this': 1, 'is': 1, 'a': 1, 'sample': 1, 'text': 1, 'it': 1, 'contains': 1, 'punctuation': 1, 'marks': 1, 'and': 1, 'we': 1, 'need': 1, 'to': 1, 'remove': 1, 'hello': 1, 'world': 1})
```
可以看到结果中没有标点符号,只有单词和它们的出现次数。
相关问题
python 词频统计 标点符号
python词频统计是一种通过统计文本中单词出现的次数来分析单词在文本中的重要性的方法。在给定的代码中,通过使用jieba库进行分词处理,然后利用字典统计每个单词出现的次数。在统计之前,代码会对文本进行预处理,去除标点符号并将文本转换为小写。最后,代码会按照单词出现的频率进行排序,并输出每个单词和对应的出现次数。
python中文词频统计,去除标点
可以使用Python的re模块和collections模块来实现中文词频统计,并去除标点符号。
以下是一个示例代码:
```python
import re
from collections import Counter
# 读取文本文件
with open('text.txt', 'r', encoding='utf-8') as f:
text = f.read()
# 去除标点符号
text = re.sub('[^\u4e00-\u9fa5]', '', text)
# 分词并统计词频
words = list(text)
word_count = Counter(words)
# 输出前10个出现频率最高的词语
for word, count in word_count.most_common(10):
print(word, count)
```
解释:
1. 使用`open`函数读取文本文件,以`utf-8`编码方式打开。
2. 使用正则表达式`[^\u4e00-\u9fa5]`匹配非中文字符,并使用`re.sub`将其替换为空字符串,实现去除标点符号的功能。
3. 将处理后的文本转化为字符列表,使用`collections.Counter`函数统计词频。
4. 使用`most_common`方法获取出现频率最高的前10个词语,并输出结果。
注:以上代码中的`text.txt`为要统计词频的文本文件名,需要根据实际情况进行修改。
阅读全文
相关推荐
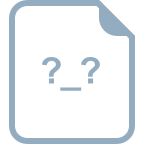
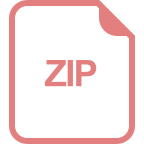
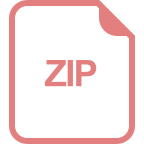
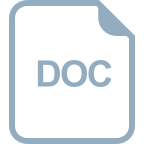
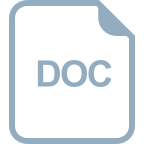





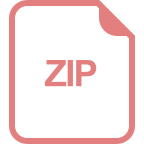
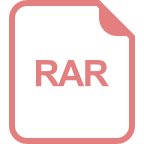


