儿童疫苗接种预约系统示例代码
时间: 2023-03-20 16:00:31 浏览: 124
对于儿童疫苗接种预约系统,需要实现以下功能:
1. 疫苗预约:父母或监护人可以选择需要接种的疫苗种类,填写儿童的基本信息,选择接种时间和地点等信息进行预约。
2. 预约查询:用户可以查询自己或者自己的孩子的预约情况,包括已经预约的疫苗种类、接种时间和地点等信息。
3. 预约取消:用户可以取消已经预约的疫苗接种。
下面是一个基于Python Flask框架的儿童疫苗接种预约系统示例代码:
```python
from flask import Flask, render_template, request, redirect, url_for
import sqlite3
app = Flask(__name__)
# 连接数据库
conn = sqlite3.connect('vaccination.db')
c = conn.cursor()
# 创建表
c.execute('CREATE TABLE IF NOT EXISTS vaccinations (id INTEGER PRIMARY KEY, '
'child_name TEXT, age INTEGER, vaccine TEXT, location TEXT, date TEXT, status TEXT)')
# 预约页面
@app.route('/', methods=['GET', 'POST'])
def appointment():
if request.method == 'POST':
child_name = request.form['child_name']
age = request.form['age']
vaccine = request.form['vaccine']
location = request.form['location']
date = request.form['date']
status = 'Pending'
# 将预约信息插入数据库
c.execute('INSERT INTO vaccinations (child_name, age, vaccine, location, date, status) '
'VALUES (?, ?, ?, ?, ?, ?)', (child_name, age, vaccine, location, date, status))
conn.commit()
return redirect(url_for('appointment'))
return render_template('appointment.html')
# 预约查询页面
@app.route('/query', methods=['GET', 'POST'])
def query():
if request.method == 'POST':
child_name = request.form['child_name']
# 从数据库中查询预约信息
c.execute('SELECT * FROM vaccinations WHERE child_name = ?', (child_name,))
vaccinations = c.fetchall()
return render_template('query_result.html', vaccinations=vaccinations)
return render_template('query.html')
# 预约取消页面
@app.route('/cancel', methods=['GET', 'POST'])
def cancel():
if request.method == 'POST':
vaccination_id = request.form['vaccination_id']
# 更新预约状态为Cancelled
c.execute('UPDATE vaccinations SET status = "Cancelled" WHERE id = ?', (vaccination_id,))
conn.commit()
return redirect(url_for('cancel'))
# 查询所有待接种的疫苗信息
c.execute('SELECT * FROM vaccinations WHERE status = "Pending"')
vaccinations = c.fetchall()
return render_template('cancel.html', vaccinations=vaccinations)
if __name__ == '__main__':
app.run(debug=True)
```
这个示例代码使用了SQLite数据库来存储预约信息。在预约页面中,用户可以填写儿童的基本信息、选择疫苗种类、接种地点和时间等信息。在预约查询页面中,用户可以通过输入儿童的姓名来查询预约信息。
阅读全文
相关推荐
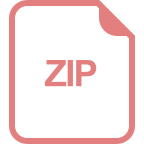
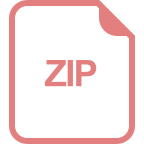
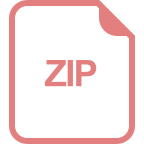
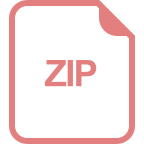
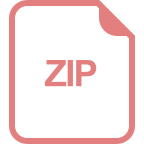
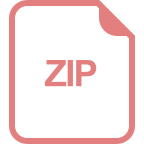
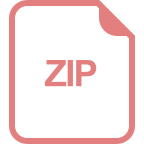
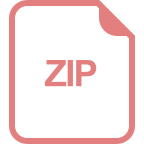
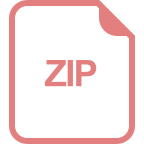
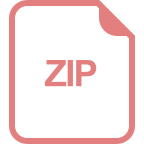
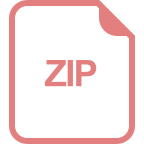
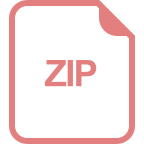
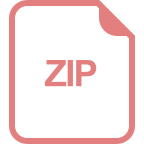
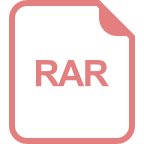
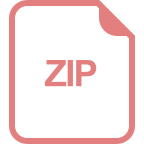
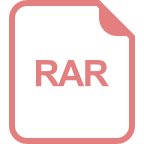
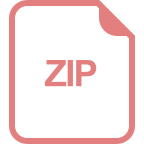