使用c语言实现简单的多任务并发程序
时间: 2024-08-14 07:02:55 浏览: 50
在 C 语言中,实现多任务并发通常不是直接的,因为 C 语言的标准库(stdio.h, stdlib.h等)并没有内置支持并发或线程的功能。然而,如果你是在一个支持多线程的平台上(如 Linux 的 pthreads 或者 Windows 的 WinAPI),你可以利用这些库来创建和管理线程。
以下是一个简单的例子,展示了如何在 Linux 平台上使用 pthread 创建两个子线程并执行各自的函数:
```c
#include <pthread.h>
#include <stdio.h>
// 定义每个线程要执行的函数
void* thread_function(void* arg) {
int id = (int)arg;
printf("Thread %d started.\n", id);
// 这里是你线程的任务代码
sleep(2); // 模拟任务执行时间
printf("Thread %d finished.\n", id);
return NULL;
}
int main() {
pthread_t thread1, thread2; // 定义线程ID
int args[] = {0, 1}; // 给线程传递参数
// 创建并启动两个线程
if (pthread_create(&thread1, NULL, thread_function, args)) {
perror("Error creating thread 1");
return 1;
}
if (pthread_create(&thread2, NULL, thread_function, &args)) {
perror("Error creating thread 2");
return 1;
}
// 等待两个线程完成
pthread_join(thread1, NULL);
pthread_join(thread2, NULL);
printf("Main thread finished.\n");
return 0;
}
```
在这个例子中,`pthread_create` 函数用于创建新的线程,`pthread_join` 用于等待线程结束。`sleep` 函数在这里只是模拟了任务执行的时间,实际上你可以替换为实际的计算或IO操作。
相关推荐
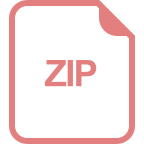
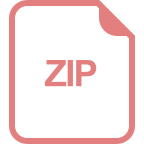
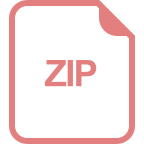














