android开发歌词滑动效果_漂亮的Android音乐歌词控件 仿网易云音乐滑动效果
时间: 2023-08-09 14:04:14 浏览: 98
您可以使用 Android 的自定义 View 来实现漂亮的歌词滑动效果。以下是一个简单的实现步骤:
1. 创建自定义 View,继承自 View 或其子类。
2. 在 onDraw() 方法中绘制歌词。
3. 在 onMeasure() 方法中计算 View 的尺寸。
4. 在 onTouchEvent() 方法中处理触摸事件,实现歌词的滑动效果。
5. 在 onDetachedFromWindow() 方法中释放资源。
以下是一个示例代码,仅供参考:
```java
public class LyricView extends View {
private static final String TAG = "LyricView";
private Paint mPaint; // 画笔
private List<String> mLyrics; // 歌词列表
private int mCurrentLine = 0; // 当前行
private int mLineHeight; // 行高
private int mMaxScroll; // 最大滚动距离
private int mScrollY; // 当前滚动距离
public LyricView(Context context) {
super(context);
init();
}
public LyricView(Context context, AttributeSet attrs) {
super(context, attrs);
init();
}
public LyricView(Context context, AttributeSet attrs, int defStyleAttr) {
super(context, attrs, defStyleAttr);
init();
}
private void init() {
mPaint = new Paint(Paint.ANTI_ALIAS_FLAG);
mPaint.setTextSize(36);
mPaint.setColor(Color.WHITE);
mPaint.setTextAlign(Paint.Align.CENTER);
mLineHeight = (int) (mPaint.getFontMetrics().bottom - mPaint.getFontMetrics().top);
}
public void setLyrics(List<String> lyrics) {
mLyrics = lyrics;
mMaxScroll = (mLyrics.size() - 1) * mLineHeight;
mCurrentLine = 0;
mScrollY = 0;
invalidate();
}
@Override
protected void onDraw(Canvas canvas) {
if (mLyrics == null || mLyrics.isEmpty()) {
return;
}
int centerY = getHeight() / 2;
for (int i = 0; i < mLyrics.size(); i++) {
String line = mLyrics.get(i);
int y = centerY + (i - mCurrentLine) * mLineHeight - mScrollY;
if (y < 0) {
continue;
}
if (y > getHeight() + mLineHeight) {
break;
}
canvas.drawText(line, getWidth() / 2, y, mPaint);
}
}
@Override
protected void onMeasure(int widthMeasureSpec, int heightMeasureSpec) {
int width = MeasureSpec.getSize(widthMeasureSpec);
int height = MeasureSpec.getSize(heightMeasureSpec);
setMeasuredDimension(width, height);
}
@Override
public boolean onTouchEvent(MotionEvent event) {
switch (event.getAction()) {
case MotionEvent.ACTION_DOWN:
// 停止自动滚动
break;
case MotionEvent.ACTION_MOVE:
int deltaY = (int) event.getY() - mLastY;
mScrollY += deltaY;
mScrollY = Math.max(0, mScrollY);
mScrollY = Math.min(mMaxScroll, mScrollY);
invalidate();
break;
case MotionEvent.ACTION_UP:
// 开始自动滚动
break;
}
mLastY = (int) event.getY();
return true;
}
@Override
protected void onDetachedFromWindow() {
super.onDetachedFromWindow();
// 释放资源
}
}
```
使用时,您可以将 LyricView 添加到您的布局文件中,并调用 setLyrics() 方法设置歌词列表即可。
阅读全文
相关推荐
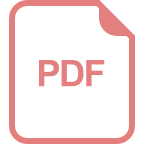
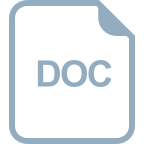
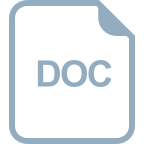
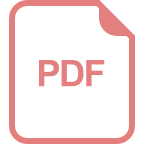
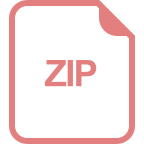
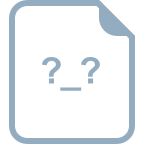
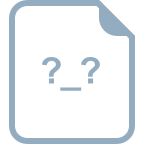
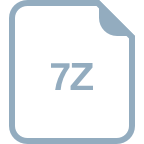
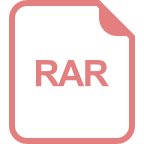
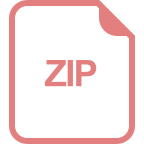
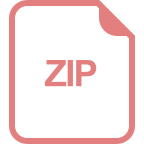
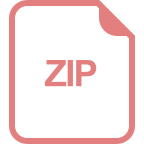
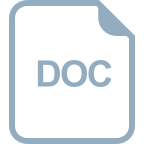
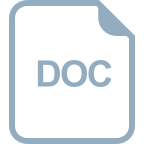
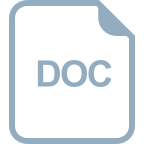