改成shape后断言正确,但是又报错,找不到索引:i = torch.sigmoid(torch.matmul(x, self.W_i_forward) + torch.matmul(h_forward_new[-1], self.U_i_forward) + self.b_i_forward) IndexError: index -1 is out of bounds for axis 0 with size 0
时间: 2024-03-03 17:51:09 浏览: 57
这个错误的原因是 `h_forward_new` 这个变量的长度为0,因此无法使用索引 -1 来获取最后一个元素。你需要确保在使用 `h_forward_new` 变量之前,它已经被正确地初始化了。另外,你还需要检查代码的逻辑是否正确,比如在某些情况下,`h_forward_new` 可能会为空,这时你需要特判处理。
另外,你可以使用 `print()` 函数来输出 `h_forward_new` 的长度或形状,以便更好地理解代码的执行过程,例如:
```python
print("h_forward_new:", h_forward_new.shape)
```
这样就可以查看 `h_forward_new` 的长度或形状了,从而更好地理解和调试代码。
相关问题
给你更多的上下文信息: for t in range(input.size(0)): x = input[t] i = torch.sigmoid(torch.matmul(x, self.W_i_forward) + torch.matmul(h_forward_new[-1], self.U_i_forward) + self.b_i_forward) f = torch.sigmoid(torch.matmul(x, self.W_f_forward) + torch.matmul(h_forward_new[-1], self.U_f_forward) + self.b_f_forward) c_tilde = torch.tanh(torch.matmul(x, self.W_c_forward) + torch.matmul(h_forward_new[-1], self.U_c_forward) + self.b_c_forward) c_forward_new[-1] = f * c_forward_new[-1] + i * c_tilde o = torch.matmul(x, self.W_o_forward) + torch.matmul(h_forward_new[-1], self.U_o_forward) + self.b_o_forward o = torch.sigmoid(o) h_forward_new[-1] = o * torch.tanh(c_forward_new[-1]) outputs_forward.append(h_forward_new[-1])
根据你提供的代码,我认为报错是因为在进行`c_forward_new[-1] = f * c_forward_new[-1] + i * c_tilde`操作时,`c_forward_new[-1]`的大小和`f * c_forward_new[-1] + i * c_tilde`的大小不一致,导致无法进行赋值操作。具体来说,可能是`c_forward_new[-1]`的大小为`[16]`,而`f * c_forward_new[-1] + i * c_tilde`的大小为`[64, 16]`。
解决这个问题的方法是,先将`c_forward_new[-1]`的大小扩展为`[64, 16]`,然后再进行赋值操作。具体代码如下:
```
c_forward_new[-1] = c_forward_new[-1].expand_as(f * c_forward_new[-1]) # 将c_forward_new[-1]扩展为与f * c_forward_new[-1]相同的大小
c_forward_new[-1] = f * c_forward_new[-1] + i * c_tilde # 进行赋值操作
```
这样就可以解决报错问题。
i = torch.sigmoid(torch.matmul(x, self.W_i_forward) + torch.matmul(h_forward_new[-1], self.U_i_forward) + self.b_i_forward) TypeError: matmul(): argument 'input' (position 1) must be Tensor, not numpy.ndarray
这个错误的原因是你在使用PyTorch时将Numpy数组传递给了PyTorch函数,而PyTorch函数期望的是一个Tensor对象。在这种情况下,你需要将Numpy数组转换为PyTorch Tensor。你可以使用以下代码将Numpy数组转换为PyTorch Tensor:
```
import torch
# 将Numpy数组转换为Tensor
x_tensor = torch.from_numpy(x_np_array)
# 使用转换后的Tensor调用PyTorch函数
i = torch.sigmoid(torch.matmul(x_tensor, self.W_i_forward) + torch.matmul(h_forward_new[-1], self.U_i_forward) + self.b_i_forward)
```
这应该可以解决你的问题。如果还有其他问题,请随时问我。
阅读全文
相关推荐
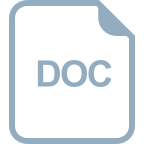
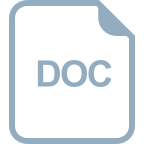
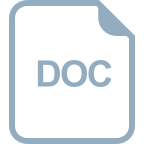
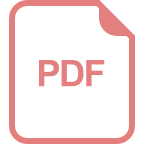
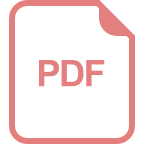
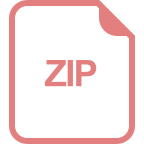
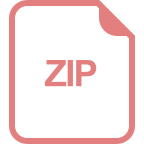
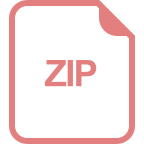
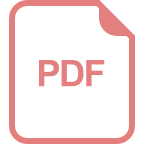
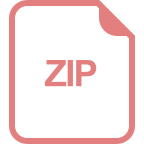
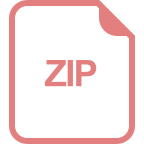
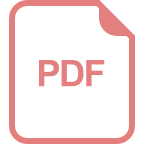
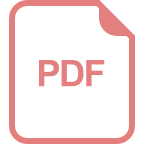
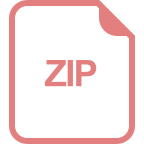
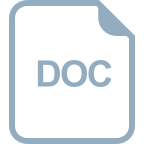