请用C++代码实现一个谓词函数
时间: 2024-03-21 15:43:02 浏览: 58
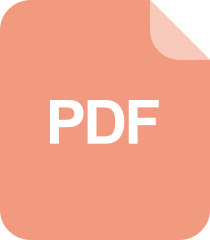
C++STL学习笔记.pdf
下面是一个简单的 C++ 谓词函数的例子,它判断一个整数是否为偶数:
```cpp
#include <iostream>
#include <vector>
#include <algorithm>
bool isEven(int n) {
return n % 2 == 0;
}
int main() {
std::vector<int> nums = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
// 使用 std::count_if 算法函数和谓词函数 isEven 统计偶数个数
int count = std::count_if(nums.begin(), nums.end(), isEven);
std::cout << "There are " << count << " even numbers." << std::endl;
return 0;
}
```
在上面的代码中,isEven 函数是一个谓词函数,它接受一个整数参数 n,返回一个布尔值,表示 n 是否为偶数。在主函数中,使用 std::count_if 算法函数和谓词函数 isEven 统计 nums 容器中偶数的个数,并输出结果。
阅读全文
相关推荐
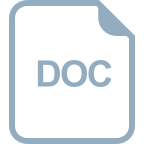
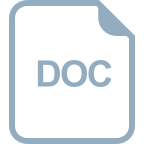
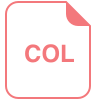



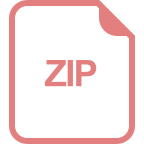
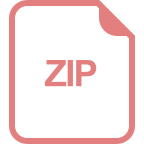
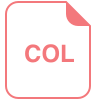
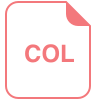




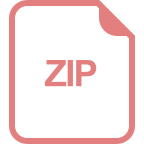
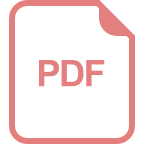
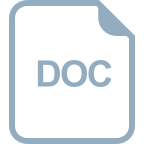
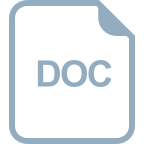