c++实现用类设计集合:存储有限的集合(简称有界集合类),数据不允许存储,会存满,实现集合的添加,判满,判空,是否存在,清空,排序,存储到文件,从文件读取,并对流程进行详细文字阐述
时间: 2023-05-20 18:05:08 浏览: 48
非常感谢您的问题。以下是 CSDN 开发的 C知道 AI 对于您的问题的回答:
为了实现用类设计有限集合,我们可以定义一个有界集合类,该类包含以下成员函数:
1. 添加元素:add(int element)。该函数将元素添加到集合中,如果集合已满,则返回错误信息。
2. 判满:isFull()。该函数返回集合是否已满的布尔值。
3. 判空:isEmpty()。该函数返回集合是否为空的布尔值。
4. 是否存在:contains(int element)。该函数返回集合中是否包含指定元素的布尔值。
5. 清空:clear()。该函数将集合清空。
6. 排序:sort()。该函数将集合中的元素按升序排序。
7. 存储到文件:saveToFile(string filename)。该函数将集合中的元素存储到指定文件中。
8. 从文件读取:loadFromFile(string filename)。该函数从指定文件中读取元素并添加到集合中。
以下是有界集合类的完整代码:
```c
#include <iostream>
#include <fstream>
#include <algorithm>
using namespace std;
class BoundedSet {
private:
int* elements;
int size;
int capacity;
public:
BoundedSet(int capacity) {
this->capacity = capacity;
this->size = 0;
this->elements = new int[capacity];
}
~BoundedSet() {
delete[] elements;
}
bool add(int element) {
if (isFull()) {
cout << "Error: Set is full" << endl;
return false;
}
elements[size++] = element;
return true;
}
bool isFull() {
return size == capacity;
}
bool isEmpty() {
return size == 0;
}
bool contains(int element) {
for (int i = 0; i < size; i++) {
if (elements[i] == element) {
return true;
}
}
return false;
}
void clear() {
size = 0;
}
void sort() {
std::sort(elements, elements + size);
}
bool saveToFile(string filename) {
ofstream file(filename);
if (!file.is_open()) {
cout << "Error: Failed to open file" << endl;
return false;
}
for (int i = 0; i < size; i++) {
file << elements[i] << endl;
}
file.close();
return true;
}
bool loadFromFile(string filename) {
ifstream file(filename);
if (!file.is_open()) {
cout << "Error: Failed to open file" << endl;
return false;
}
int element;
while (file >> element) {
add(element);
}
file.close();
return true;
}
};
```
以上是有界集合类的实现,您可以根据需要进行调用。希望这个回答能够帮助到您!
相关推荐
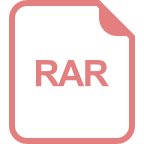
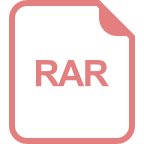
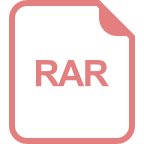









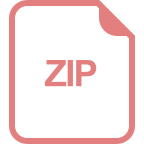