dataframe添加字典
时间: 2023-07-06 11:10:42 浏览: 58
要向 DataFrame 中添加字典数据,可以使用 `append` 方法。例如,如果您有一个 DataFrame `df`,可以将一个字典添加为一行数据,如下所示:
```
new_dict = {'col1': 1, 'col2': 2, 'col3': 3}
df = df.append(new_dict, ignore_index=True)
```
其中,`new_dict` 是要添加的字典。`ignore_index=True` 表示忽略原有的行索引,使用新的行索引。
另一种常见的方式是使用 `pandas.DataFrame.from_dict` 方法,将字典转换为 DataFrame,然后使用 `concat` 方法将其与原始 DataFrame 连接。例如:
```
new_dict = {'col1': 1, 'col2': 2, 'col3': 3}
new_df = pd.DataFrame.from_dict(new_dict, orient='index').T
df = pd.concat([df, new_df], ignore_index=True)
```
其中,`pd.DataFrame.from_dict` 方法将字典转换为 DataFrame,`orient='index'` 表示将字典的键作为列名,`T` 表示转置 DataFrame,以使其行变为列。然后使用 `concat` 方法将新的 DataFrame 与原始 DataFrame 连接。`ignore_index=True` 表示忽略原有的行索引,使用新的行索引。
相关问题
python dataframe 添加数据
在Python中,可以使用pandas库来创建和操作DataFrame。要向DataFrame中添加数据,可以使用以下方法:
1. 使用字典创建DataFrame并添加数据[^1]:
```python
import pandas as pd
# 创建空的DataFrame
df = pd.DataFrame()
# 创建字典
data = {'name': ['apple', 'egg', 'watermelon'], 'color': ['red', 'yellow', 'green'], 'num': [30, 40, 50]}
# 将字典数据添加到DataFrame中
df = df.append(data, ignore_index=True)
```
2. 使用已有的DataFrame对象添加数据[^2]:
```python
import pandas as pd
# 创建空的DataFrame
df = pd.DataFrame()
# 创建字典
data = {'name': ['apple', 'egg', 'watermelon'], 'color': ['red', 'yellow', 'green'], 'num': [30, 40, 50]}
# 创建索引和列名
index = ['a', 'b', 'c']
columns = ['name', 'color', 'num']
# 创建DataFrame并添加数据
df = pd.DataFrame(data=data, index=index, columns=columns)
```
以上是两种常用的方法来向Python DataFrame中添加数据。
dataframe添加行
在 Pandas 中,可以使用 `append()` 方法向 DataFrame 添加行。以下是一个示例:
```python
import pandas as pd
# 创建一个空的 DataFrame
df = pd.DataFrame(columns=['Name', 'Age'])
# 添加一行数据
df = df.append({'Name': 'John', 'Age': 25}, ignore_index=True)
print(df)
```
输出:
```
Name Age
0 John 25
```
在 `append()` 方法中,你可以传递一个字典或 Series 对象来表示要添加的行。`ignore_index=True` 参数用于重置索引,以便在添加行时,该行的索引自动设置为 DataFrame 中最大的索引值加1。
相关推荐
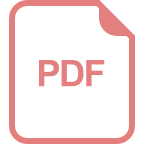












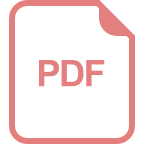