GNN-LSTM 代码
时间: 2023-10-24 09:29:01 浏览: 90
以下是使用PyTorch实现的GNN-LSTM的代码示例,其中包括了GNN和LSTM的实现:
```python
import torch
import torch.nn as nn
import torch.nn.functional as F
from torch_geometric.nn import GCNConv
class GNNLSTM(nn.Module):
def __init__(self, input_dim, hidden_dim, output_dim, num_layers, dropout):
super(GNNLSTM, self).__init__()
self.input_dim = input_dim
self.hidden_dim = hidden_dim
self.output_dim = output_dim
self.num_layers = num_layers
self.dropout = dropout
# GNN part
self.conv1 = GCNConv(input_dim, hidden_dim)
self.convs = nn.ModuleList()
for i in range(num_layers - 1):
self.convs.append(GCNConv(hidden_dim, hidden_dim))
self.conv_last = GCNConv(hidden_dim, output_dim)
# LSTM part
self.lstm = nn.LSTM(input_dim, hidden_dim, num_layers, batch_first=True, dropout=dropout)
def forward(self, x, edge_index, h, c):
# GNN part
x = F.relu(self.conv1(x, edge_index))
for conv in self.convs:
x = F.relu(conv(x, edge_index))
x = F.dropout(x, self.dropout, training=self.training)
x = self.conv_last(x, edge_index)
# LSTM part
x, (h, c) = self.lstm(x.unsqueeze(0), (h, c))
x = x.squeeze(0)
return x, h, c
```
在这个GNN-LSTM模型中,我们使用了一个GCNConv层来实现GNN的部分,使用了一个nn.LSTM层来实现LSTM的部分。在forward方法中,我们首先对输入进行GNN计算,然后将结果作为LSTM的输入,获得最终的输出。
相关推荐
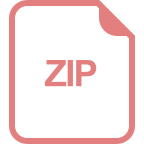
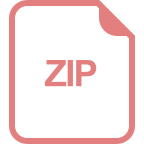
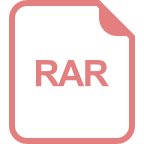














