用java编写这个程序:信息学院年终评定奖学金,需要对整个年级的学生按照平均分数进行排名。 要求:根据输入的学号和平均成绩,按照平均成绩降序输出学号,如果平均成绩相同,按照输入的顺序输出。
时间: 2024-12-15 15:24:43 浏览: 14
在Java中,你可以创建一个`Student`类来存储学生的信息,包括学号和平均分数。然后,可以使用`PriorityQueue`数据结构,因为它是自调整大小的最小堆,用于存储学生,并保证按平均分数从大到小排序。这里是一个简单的示例:
```java
import java.util.*;
class Student implements Comparable<Student> {
String id;
double averageScore;
// 构造函数
public Student(String id, double averageScore) {
this.id = id;
this.averageScore = averageScore;
}
@Override
public int compareTo(Student other) {
if (this.averageScore == other.averageScore) {
return Integer.compare(this.id, other.id);
}
return Double.compare(this.averageScore, other.averageScore); // 降序
}
@Override
public String toString() {
return "ID: " + id + ", Average Score: " + averageScore;
}
}
public class ScholarshipRanking {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
PriorityQueue<Student> queue = new PriorityQueue<>((a, b) -> -b.compareTo(a)); // 降序
System.out.println("Enter student ID and average score, separated by space:");
while (scanner.hasNext()) {
String[] input = scanner.nextLine().split(" ");
queue.offer(new Student(input[0], Double.parseDouble(input[1])));
}
// 输出排名
while (!queue.isEmpty()) {
System.out.println(queue.poll());
}
scanner.close();
}
}
```
在这个程序中,用户通过命令行依次输入学号和平均分数,程序会实时添加到队列中并保持按得分降序排列。当队列为空时,说明所有学生已经处理完毕。
阅读全文
相关推荐
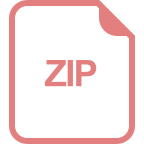














