通过键盘录入员工年终绩效信息,写到绩效文件中,然后从员工绩效文件中个读取绩效数据进行分析,显示最高绩效、最低绩效和平均绩效和总绩效。用Java实现
时间: 2024-12-20 11:30:49 浏览: 9
在Java中实现这个功能,你可以按照以下几个步骤操作:
1. **设计员工类(Employee)**: 定义一个Employee类,包含姓名(name)、岗位(position)和年终绩效(annualPerformance)等属性。
```java
public class Employee {
private String name;
private String position;
private double annualPerformance;
// 构造函数、getter和setter方法...
}
```
2. **创建绩效文件(PerformanceFile)**: 使用ArrayList或List接口存储Employee对象,模拟文件。
```java
import java.util.List;
public class PerformanceFile {
private List<Employee> employees;
public PerformanceFile() {
this.employees = new ArrayList<>();
}
// 添加员工、读取数据的方法...
}
```
3. **录入绩效信息**: 创建键盘录入方法,让用户输入员工信息并添加到绩效文件中。
```java
public void addEmployee() {
Scanner scanner = new Scanner(System.in);
System.out.println("请输入员工信息:");
String name = scanner.nextLine();
String position = scanner.nextLine();
double performance = scanner.nextDouble(); // 输入年度绩效
Employee employee = new Employee(name, position, performance);
employees.add(employee);
}
```
4. **读取并分析数据**: 提供方法计算最高、最低、平均绩效以及总绩效。
```java
public void analyzePerformance() {
if (employees.isEmpty()) {
System.out.println("文件为空");
} else {
double maxPerformance = employees.get(0).getAnnualPerformance();
double minPerformance = employees.get(0).getAnnualPerformance();
for (Employee e : employees) {
maxPerformance = Math.max(maxPerformance, e.getAnnualPerformance());
minPerformance = Math.min(minPerformance, e.getAnnualPerformance());
}
double average = employees.stream().mapToDouble(Employee::getAnnualPerformance).average().orElse(Double.NaN);
double total = employees.stream().mapToDouble(Employee::getAnnualPerformance).sum();
System.out.printf("最高绩效: %.2f%n", maxPerformance);
System.out.printf("最低绩效: %.2f%n", minPerformance);
System.out.printf("平均绩效: %.2f%n", average);
System.out.printf("总绩效: %.2f%n", total);
}
}
```
5. **主程序循环**: 可以设置一个简单的循环,允许用户多次录入和分析性能。
```java
public static void main(String[] args) {
PerformanceFile pf = new PerformanceFile();
while (true) {
System.out.println("请选择操作: 1.录入 2.分析 3.退出");
int choice = scanner.nextInt();
switch (choice) {
case 1: pf.addEmployee(); break;
case 2: pf.analyzePerformance(); break;
case 3: return; // 退出
default: System.out.println("无效选择,请重试");
}
}
}
```
阅读全文
相关推荐
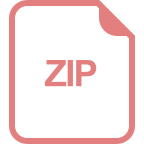
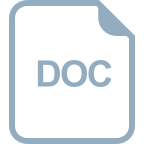
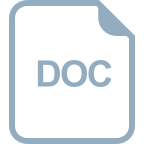

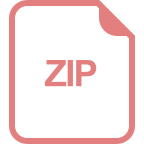
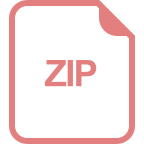
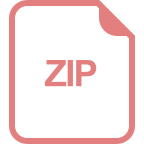
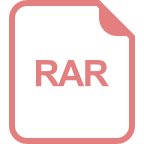
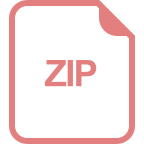
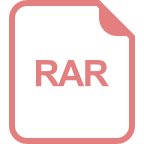
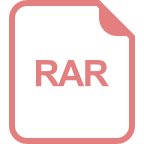
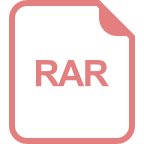
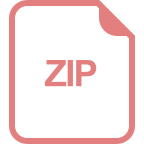
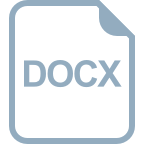
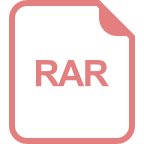
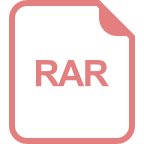
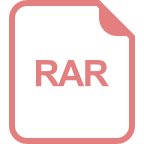
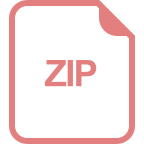
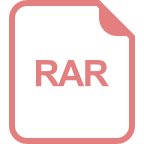