程序首先打印出主线程的ID然后打印新创建线程的ID代码
时间: 2023-12-18 19:04:46 浏览: 106
好的,以下是一个示例代码,可以打印出主线程的ID和新创建线程的ID:
```python
import threading
def new_thread():
print("New thread ID:", threading.get_ident())
print("Main thread ID:", threading.get_ident())
t = threading.Thread(target=new_thread)
t.start()
```
运行这段代码后,会先打印出主线程的ID,然后再打印出新创建线程的ID。注意,每次运行程序时,新创建线程的ID都是随机生成的,因此输出结果可能不同。
相关问题
程序首先打印出主线程的ID然后打印新创建线程的IDC语言代码
### 回答1:
下面是一个简单的 C 语言示例代码,可以打印出主线程的 ID 和新创建线程的 ID:
```c
#include <stdio.h>
#include <pthread.h>
void* thread_func(void* arg)
{
printf("新创建的线程的 ID 是:%lu\n", pthread_self());
return NULL;
}
int main()
{
printf("主线程的 ID 是:%lu\n", pthread_self());
pthread_t thread;
int ret = pthread_create(&thread, NULL, thread_func, NULL);
if (ret != 0) {
printf("创建线程失败\n");
return 1;
}
pthread_join(thread, NULL);
return 0;
}
```
程序首先打印出主线程的 ID,然后创建一个新的线程,并打印出新创建线程的 ID。最后通过 `pthread_join` 函数等待新线程结束,以确保程序正常退出。
### 回答2:
在C语言中,我们可以使用`pthread_create()`函数来创建新的线程。
```c
#include <stdio.h>
#include <pthread.h>
void* thread_function(void* arg)
{
printf("新线程的ID:%lu\n", pthread_self());
pthread_exit(NULL);
}
int main()
{
printf("主线程的ID:%lu\n", pthread_self());
pthread_t thread;
pthread_create(&thread, NULL, thread_function, NULL);
pthread_join(thread, NULL); // 等待新线程执行完毕
return 0;
}
```
在主函数中,首先打印出主线程的ID,使用`pthread_self()`函数获取当前线程的ID,并通过`%lu`格式化输出。
接下来,使用`pthread_create()`函数创建新的线程,第一个参数是指向线程标识符的指针,第二个参数用于设置线程的属性,第三个参数是新线程要执行的函数,最后一个参数是传递给新线程的参数。在上述代码中,`thread_function()`函数用于新线程的执行,参数为`NULL`。
最后,使用`pthread_join()`函数在主线程中等待新线程的执行完成。最后返回0结束程序的执行。
当程序运行时,首先打印出主线程的ID,然后创建新线程,并打印出新线程的ID。
### 回答3:
我们可以使用C语言中的线程库pthread来创建和管理线程。以下是一个示例代码,实现了在主线程中打印出主线程ID,然后创建一个新线程并打印出其ID的功能。
```c
#include <stdio.h>
#include <pthread.h>
void* printThreadID(void* arg) {
// 打印新线程的ID
printf("新线程的ID:%lu\n", pthread_self());
pthread_exit(NULL);
}
int main() {
// 打印主线程的ID
printf("主线程的ID:%lu\n", pthread_self());
pthread_t thread;
int result = pthread_create(&thread, NULL, printThreadID, NULL);
if (result != 0) {
printf("创建新线程失败\n");
return 1;
}
pthread_join(thread, NULL); // 等待新线程结束
return 0;
}
```
运行代码后,会先打印出主线程的ID,然后创建一个新线程并打印出其ID。
编写一个c程序,在主线程中创建4个新线程,所有线程都输出自己的线程号
好的,下面是代码:
```c
#include <stdio.h>
#include <pthread.h>
void *print_thread_id(void *arg) {
printf("Thread ID: %ld\n", (long)arg);
pthread_exit(NULL);
}
int main() {
pthread_t threads[4];
int i;
for (i = 0; i < 4; i++) {
if (pthread_create(&threads[i], NULL, print_thread_id, (void *)i) != 0) {
fprintf(stderr, "Error creating thread\n");
return -1;
}
}
for (i = 0; i < 4; i++) {
if (pthread_join(threads[i], NULL) != 0) {
fprintf(stderr, "Error joining thread\n");
return -1;
}
}
return 0;
}
```
这个程序创建了4个新线程,每个线程都输出自己的线程号。在主线程中,我们使用 `pthread_create()` 函数创建新线程,然后使用 `pthread_join()` 函数等待所有线程结束。
阅读全文
相关推荐
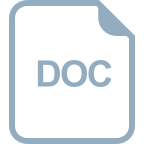
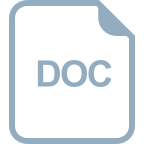
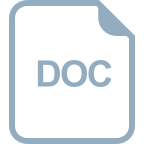












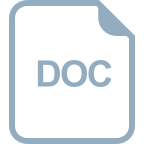
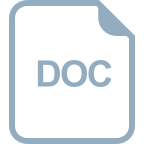