举一个c++ list插入元素的例子
时间: 2023-08-10 14:05:14 浏览: 49
下面是一个简单的例子,演示如何使用C++的list容器插入元素:
```c++
#include <iostream>
#include <list>
using namespace std;
int main() {
list<int> myList; // 定义一个空的 list
myList.push_back(10); // 在 list 尾部插入一个元素 10
myList.push_back(20); // 在 list 尾部插入一个元素 20
myList.push_front(30); // 在 list 头部插入一个元素 30
// 在 list 中间插入一个元素 15,使用 insert() 方法
list<int>::iterator it = myList.begin();
it++; // 指向第二个元素
myList.insert(it, 15);
// 输出 list 中所有元素
for (auto i : myList) {
cout << i << " ";
}
cout << endl;
return 0;
}
```
输出结果为:`30 15 10 20`。
相关问题
c++list删除元素
在C++中,可以使用list容器的erase()方法来删除元素。以下是一个例子:
```c++
#include <iostream>
#include <list>
using namespace std;
int main() {
// 初始化一个int的list
list<int> intList;
for (int i = 0; i <= 3; i++) {
intList.push_back(i);
}
// 使用push_back向List加内容
list<int>::iterator itor; // 声明一个Int的List的迭代器
for (itor = intList.begin(); itor != intList.end(); itor++) {
if (*itor == 2) {
itor = intList.erase(itor); // 删除元素
}
}
// 输出删除后的list
for (itor = intList.begin(); itor != intList.end(); itor++) {
cout << *itor << " ";
}
cout << endl;
return 0;
}
```
上述代码中,我们首先初始化了一个int类型的list,然后使用push_back()方法向list中添加元素。接着,我们使用迭代器遍历list,当遍历到值为2的元素时,使用erase()方法删除该元素。最后,我们再次使用迭代器遍历list,输出删除后的结果。
c++中怎么写一个list,元素为任何类型
在C语言中,没有内置的list数据结构。但是我们可以通过自定义结构体和指针来实现一个类似于list的数据结构。
以下是一个简单的例子:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct node {
void *data;
struct node *next;
} Node;
typedef struct {
Node *head;
Node *tail;
int size;
} List;
List *list_create() {
List *list = malloc(sizeof(List));
list->head = NULL;
list->tail = NULL;
list->size = 0;
return list;
}
void list_add(List *list, void *data) {
Node *node = malloc(sizeof(Node));
node->data = data;
node->next = NULL;
if (list->head == NULL) {
list->head = node;
} else {
list->tail->next = node;
}
list->tail = node;
list->size++;
}
void list_remove(List *list, int index) {
if (index < 0 || index >= list->size) {
return;
}
Node *node = list->head;
Node *prev = NULL;
for (int i = 0; i < index; i++) {
prev = node;
node = node->next;
}
if (prev == NULL) {
list->head = node->next;
} else {
prev->next = node->next;
}
if (node == list->tail) {
list->tail = prev;
}
free(node);
list->size--;
}
void list_print(List *list) {
Node *node = list->head;
while (node != NULL) {
printf("%p\n", node->data);
node = node->next;
}
}
void list_destroy(List *list) {
Node *node = list->head;
while (node != NULL) {
Node *next = node->next;
free(node);
node = next;
}
free(list);
}
int main() {
List *list = list_create();
int a = 1;
int b = 2;
float c = 3.14;
char *d = "hello";
list_add(list, &a);
list_add(list, &b);
list_add(list, &c);
list_add(list, d);
list_print(list);
list_remove(list, 2);
list_print(list);
list_destroy(list);
return 0;
}
```
在上面的代码中,我们定义了一个结构体`Node`,它包含一个指向任何类型数据的指针`data`和一个指向下一个节点的指针`next`。我们还定义了一个结构体`List`,它包含链表的头和尾节点指针,以及链表的大小。
`list_create`函数用于创建一个空的链表。`list_add`函数用于向链表中添加一个元素,它接受一个指向任何类型数据的指针作为参数。`list_remove`函数用于从链表中删除指定位置的元素。`list_print`函数用于打印链表中所有元素的地址。`list_destroy`函数用于释放链表占用的内存。
在主函数中,我们向链表中添加了四个元素:一个整数、一个浮点数、一个字符串和另一个整数。然后我们打印了链表中所有元素的地址,并删除了第三个元素。最后,我们释放了链表占用的内存。
需要注意的是,由于我们使用了指向任何类型数据的指针,因此在使用时需要注意数据类型的匹配和转换。
相关推荐
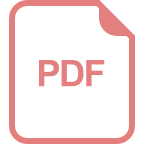












