可以用C#写一个入库程序,扫描指定xlsx表格内容分配导入指定数据库吗
时间: 2024-03-05 07:48:58 浏览: 59
可以的。你可以使用 C# 中的 OpenXML 库来读取和解析 Excel 文件。然后,你可以使用 ADO.NET 或 Entity Framework 等技术来连接和操作数据库。
以下是一个实现的示例代码:
```csharp
using System;
using System.Data;
using System.Data.SqlClient;
using DocumentFormat.OpenXml.Packaging;
using DocumentFormat.OpenXml.Spreadsheet;
public void ImportDataFromExcel(string filePath, string connectionString)
{
// Open the Excel file using OpenXML library.
using (SpreadsheetDocument doc = SpreadsheetDocument.Open(filePath, false))
{
// Read the first sheet from the workbook.
WorksheetPart worksheetPart = doc.WorkbookPart.WorksheetParts.First();
Worksheet worksheet = worksheetPart.Worksheet;
SheetData sheetData = worksheet.GetFirstChild<SheetData>();
// Connect to the database.
using (SqlConnection connection = new SqlConnection(connectionString))
{
connection.Open();
// Iterate through the rows in the sheet.
foreach (Row row in sheetData.Descendants<Row>())
{
// Skip the header row.
if (row.RowIndex.Value == 1)
{
continue;
}
// Read the values from the cells in the row.
string column1Value = GetCellValue(doc, row.Descendants<Cell>().ElementAt(0));
string column2Value = GetCellValue(doc, row.Descendants<Cell>().ElementAt(1));
string column3Value = GetCellValue(doc, row.Descendants<Cell>().ElementAt(2));
// Insert the data into the database.
SqlCommand command = new SqlCommand("INSERT INTO MyTable (Column1, Column2, Column3) VALUES (@Column1, @Column2, @Column3)", connection);
command.Parameters.AddWithValue("@Column1", column1Value);
command.Parameters.AddWithValue("@Column2", column2Value);
command.Parameters.AddWithValue("@Column3", column3Value);
command.ExecuteNonQuery();
}
connection.Close();
}
}
}
private string GetCellValue(SpreadsheetDocument doc, Cell cell)
{
SharedStringTablePart stringTablePart = doc.WorkbookPart.SharedStringTablePart;
if (cell.DataType != null && cell.DataType.Value == CellValues.SharedString)
{
return stringTablePart.SharedStringTable.ChildElements[int.Parse(cell.CellValue.InnerText)].InnerText;
}
else
{
return cell.CellValue.InnerText;
}
}
```
这个代码会读取指定的 Excel 文件,然后将数据插入到名为 `MyTable` 的数据库表中。你需要将 `connectionString` 参数替换为你的数据库连接字符串,以及根据你的 Excel 文件和数据库表结构修改代码。
阅读全文
相关推荐
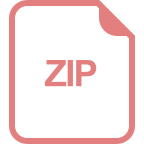
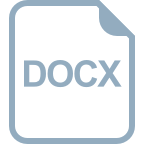
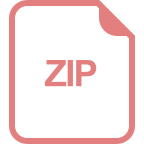
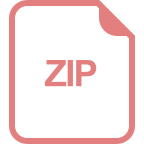
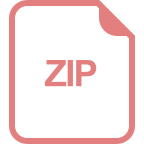
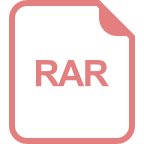