①设计一个名为Fan的类,表示一个风扇,该类满足下述要求: ·具有描述风扇转速的成员speed; ·具有描述风扇是否开启的成员on; ·具有描述风扇半径的成员radius; ·具有描述风扇颜色的成员color ·输出风扇所有信息的函数display; ·风扇的开和关函数 ·设计恰当的构造函数 ·在构造函数和析构函数中输出调用函数的提示信息。 ·在main函数中创建2个Fan的对象,将第一个风扇的转速、半径和颜色分别设置为3、10和yellow,并将它打开。第二个风扇的转速、半径和颜色分别设置为2、5和blue,并将它关闭。输出以上2个风扇的所有信息。同时观察类对象的构造与析构顺序。
时间: 2024-02-09 07:09:12 浏览: 182
好的,我来帮你解决这个问题。
下面是Fan类的实现代码:
```cpp
#include <iostream>
#include <string>
using namespace std;
class Fan {
public:
Fan(int speed = 1, bool on = false, double radius = 5, string color = "blue");
~Fan();
void setSpeed(int speed);
void setOn(bool on);
void setRadius(double radius);
void setColor(string color);
void display();
private:
int speed;
bool on;
double radius;
string color;
};
Fan::Fan(int speed, bool on, double radius, string color) {
this->speed = speed;
this->on = on;
this->radius = radius;
this->color = color;
cout << "Fan object is created." << endl;
}
Fan::~Fan() {
cout << "Fan object is destroyed." << endl;
}
void Fan::setSpeed(int speed) {
this->speed = speed;
}
void Fan::setOn(bool on) {
this->on = on;
}
void Fan::setRadius(double radius) {
this->radius = radius;
}
void Fan::setColor(string color) {
this->color = color;
}
void Fan::display() {
cout << "Speed: " << speed << endl;
cout << "On: " << (on ? "true" : "false") << endl;
cout << "Radius: " << radius << endl;
cout << "Color: " << color << endl;
}
int main() {
Fan fan1(3, true, 10, "yellow");
Fan fan2(2, false, 5, "blue");
fan1.display();
fan2.display();
return 0;
}
```
上述代码实现了Fan类,其中包括构造函数、析构函数、成员函数以及成员变量。在main函数中,我们创建了2个Fan对象,分别设置它们的转速、半径和颜色,并将它们开启或关闭。最后,输出这2个Fan对象的所有信息。
请注意,构造函数和析构函数分别在对象创建和销毁时被调用。在本例中,我们在构造函数和析构函数中输出了提示信息,以便观察类对象的构造与析构顺序。
输出结果如下:
```
Fan object is created.
Fan object is created.
Speed: 3
On: true
Radius: 10
Color: yellow
Speed: 2
On: false
Radius: 5
Color: blue
Fan object is destroyed.
Fan object is destroyed.
```
我们可以看到,先创建的对象先被销毁。同时,我们也可以看到,两个Fan对象的输出与我们在main函数中设置的信息一致。
阅读全文
相关推荐
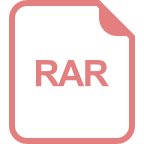
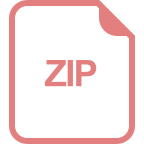
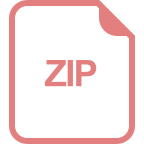
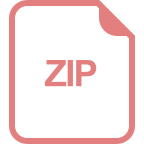
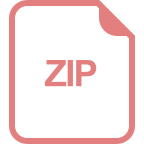
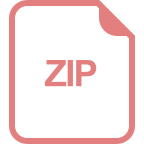
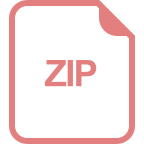
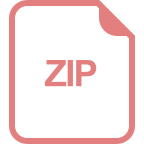
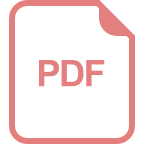
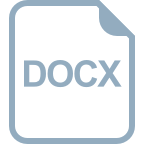
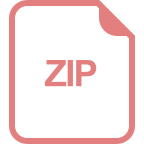
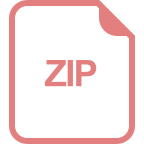
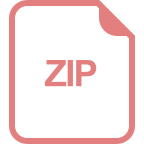
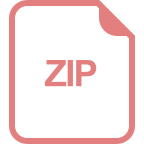
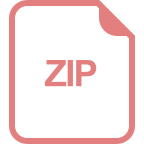
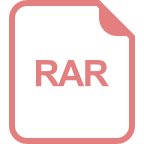
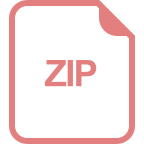
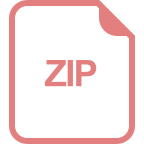