在vue中怎么通过路由拿到id
时间: 2024-02-20 07:56:48 浏览: 125
在 Vue 中,我们可以使用 Vue Router 来进行路由管理。如果你想要通过路由获取传入的 id 参数,可以在路由配置的时候进行配置。假设你的路由是这样的:
```javascript
const router = new VueRouter({
routes: [
{
path: '/user/:id',
name: 'User',
component: User
}
]
})
```
在这个路由中,我们使用了 `:id` 来表示传入的 id 参数。然后,在 `User` 组件中,我们可以通过 `this.$route.params.id` 来获取传入的 id 参数。例如:
```javascript
<template>
<div>
<h1>User ID: {{ userId }}</h1>
</div>
</template>
<script>
export default {
computed: {
userId() {
return this.$route.params.id
}
}
}
</script>
```
在这个例子中,我们使用了计算属性 `userId` 来获取传入的 id 参数,并在模板中显示出来。
相关问题
vue3路由配置文件中怎么拿到pinia里的值
在Vue 3中,如果你想要在路由配置文件中获取Pinia库中的状态值,你需要遵循这样的步骤:
1. **设置Pinia Store**: 首先,在你的应用里创建一个Pinia store,并保存你想要在路由中访问的数据。例如,假设你在store.js里有一个名为`myData`的状态:
```javascript
import { createStore } from 'pinia';
export const useMyData = createStore({
id: 'myData',
state: () => ({
myValue: 'default value'
}),
getters: {
getMyValue() {
return this.myValue;
}
},
});
```
2. **注入store到组件**: 确保在需要使用这个数据的组件中通过`provide`和`inject`来注入store:
```javascript
import { inject } from 'vue';
import { useMyData } from '@/store';
export default {
setup() {
const myData = inject('myData');
// 使用getMyValue方法获取store中的值
const currentValue = myData.getMyValue();
// ...
return {
currentValue,
};
},
};
```
3. **在路由钩子中使用**: 如果你希望在路由导航之前或之后获取并处理store值,可以考虑在`beforeRouteEnter`或`beforeRouteUpdate`等生命周期钩子里做这件事。然而,记住,一般情况下,我们不会直接在路由配置文件中操作store,因为这通常会使代码变得复杂且不易维护。
```javascript
import { beforeRouteEnter } from 'vue-router';
// ...
router.beforeEach((to, from, next) => {
const MyComponent = resolve => require(['@/components/MyComponent.vue'], resolve);
beforeRouteEnter(to.meta, to, component => {
component(currentContext => {
// 当前组件上下文 context,包含 props 和 inject 注入的 store
const myData = currentContext injections.myData; // 如果在setup中注入了别名
// ...
next(); // 继续执行路由跳转
});
});
});
vue3项目调一个鉴权接口 这个接口会返回一个业务员的工号 用pinia想把这个工号存起来 让每个组件调接口的时候都可以随时拿到这个工号 怎么做
在 Vue3 中,可以使用 Pinia 存储管理状态(类似于 Vuex 的 state),来保存全局共享的数据,比如业务员的工号。以下是步骤:
1. 安装并配置 Pinia:首先安装 `@vue/pinia` 和 `vue-router-pwa`(如果需要路由守卫),然后创建一个 store 文件,例如 `store/auth.js`,用于存放工号信息。
```javascript
import { defineStore } from 'pinia';
export const useEmployeeIdStore = defineStore('employeeId', {
state: () => ({
employeeId: null, // 初始值设为 null
}),
actions: {
async fetchEmployeeId() {
try {
const response = await axios.get('/auth'); // 调用鉴权接口
this.employeeId = response.data.id; // 存放工号
} catch (error) {
console.error('Error fetching employee ID:', error);
}
},
},
});
```
2. 在 `App.vue` 或者其他合适的地方初始化 store 并触发获取工号操作:
```javascript
import { useEmployeeIdStore } from '@/store/auth';
setup() {
const store = useEmployeeIdStore();
onMounted(() => {
store.fetchEmployeeId(); // 首次加载页面时获取工号
});
return {
//...
};
}
```
3. 现在在任何组件中,你可以通过注入 `useEmployeeIdStore` 来访问工号:
```javascript
import { computed } from 'vue';
import { useEmployeeIdStore } from '@/store/auth';
setup() {
const store = useEmployeeIdStore();
const employeeId = computed(() => store.employeeId);
return {
employeeId,
//...
};
}
// 组件内的模板里就可以直接使用 `this.employeeId`
```
阅读全文
相关推荐
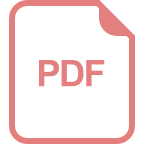
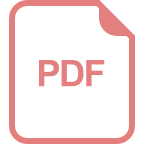
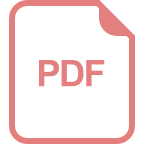
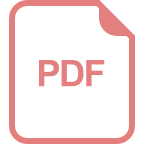
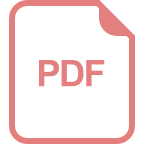
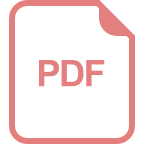
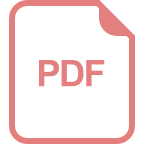
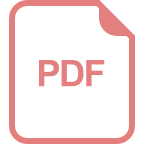
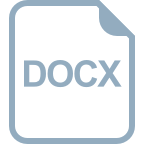
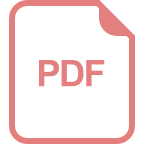
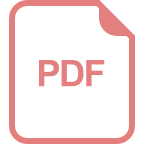
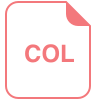



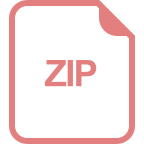