假设电子通讯录包含姓名、部门、年龄、电话等信息,采用记事本存储具体信息,首先,从记事本中依次读取每一行通讯录内容,将读取的所有教师信息按照年龄构建成二叉排序树,
时间: 2023-08-24 13:07:15 浏览: 80
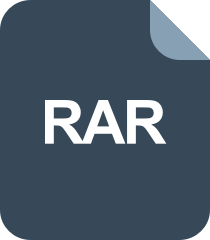
用c实现的二叉树的中序遍历的读取与存储,能体现出向左倒的二叉树的打印界面,界面简单.rar
好的,我们可以按照以下步骤来实现:
1. 定义一个教师信息的数据结构,包含姓名、部门、年龄、电话等字段。
2. 从记事本中依次读取每一行通讯录内容,并将其转换为教师信息的数据结构。
3. 将所有教师信息按照年龄升序排序。
4. 以排序后的第一个教师信息为根节点,创建一个二叉排序树。
5. 遍历排序后的其他教师信息,依次插入到二叉排序树中。
下面是一个基于 Python 的实现示例:
```python
# 教师信息数据结构
class Teacher:
def __init__(self, name, department, age, phone):
self.name = name
self.department = department
self.age = age
self.phone = phone
# 读取通讯录文件
def read_contacts(filename):
contacts = []
with open(filename, 'r') as f:
for line in f:
fields = line.strip().split('\t')
name, department, age, phone = fields[0], fields[1], int(fields[2]), fields[3]
teacher = Teacher(name, department, age, phone)
contacts.append(teacher)
return contacts
# 创建二叉排序树节点
class TreeNode:
def __init__(self, teacher):
self.teacher = teacher
self.left = None
self.right = None
# 插入教师信息到二叉排序树
def insert_node(root, teacher):
if root is None:
return TreeNode(teacher)
if teacher.age < root.teacher.age:
root.left = insert_node(root.left, teacher)
else:
root.right = insert_node(root.right, teacher)
return root
# 构建二叉排序树
def build_tree(contacts):
root = None
for teacher in contacts:
if root is None:
root = TreeNode(teacher)
else:
root = insert_node(root, teacher)
return root
# 中序遍历二叉排序树
def inorder_traversal(root):
if root is not None:
inorder_traversal(root.left)
print(root.teacher.name, root.teacher.age)
inorder_traversal(root.right)
# 主程序入口
if __name__ == '__main__':
# 读取通讯录文件
contacts = read_contacts('contacts.txt')
# 按照年龄升序排序
contacts.sort(key=lambda x: x.age)
# 构建二叉排序树
root = build_tree(contacts)
# 中序遍历二叉排序树
inorder_traversal(root)
```
以上示例中,我们首先定义了一个教师信息的数据结构,并编写了读取通讯录文件的函数,将每一行通讯录内容转换为教师信息的数据结构。
然后,我们以排序后的第一个教师信息为根节点,创建了一个二叉排序树,遍历排序后的其他教师信息,依次插入到二叉排序树中。
最后,我们通过中序遍历二叉排序树,将所有教师信息按照年龄升序输出。
阅读全文
相关推荐










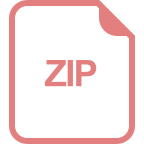
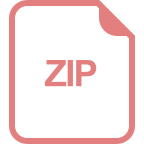
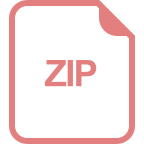
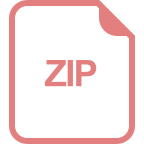
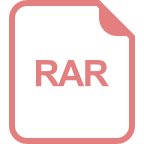
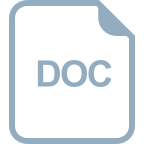