假设电子通讯录包含姓名、部门、年龄、电话等信息,采用记事本存储具体信息 首先,从记事本中依次读取每一行通讯录内容,将读取的所有教师信息按照年龄构建成二叉排序树,如图8-2所示。然后,分别通过对二叉排序树的先序遍历显示所有教师信息,通过二叉排序树的后序遍历按照姓名查询某位教师通讯信息,通过二叉排序树后序遍历按照部门查询该部门所有教师的通讯信息;最后,通过二叉排序树的中序遍历输出青年教师(≤35岁)通讯信息,遍历过程中,如果某个结点的年龄大于35岁,那么不再需要遍历该结点的右子树。 】
时间: 2024-03-01 15:50:16 浏览: 72
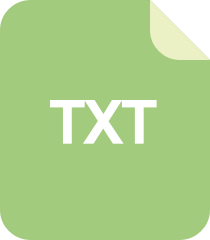
基于二叉排序树写的通讯录
好的,以下是一段简单的代码实现,注释已经添加在代码后面:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义通讯录结构体
typedef struct {
char name[20];
char department[20];
int age;
char phone[20];
} Contact;
// 定义二叉排序树结构体
typedef struct TreeNode {
Contact data;
struct TreeNode *left;
struct TreeNode *right;
} TreeNode, *Tree;
// 从文件中读取通讯录信息
void readFromFile(char *filename, Contact *contacts, int *n) {
FILE *fp;
fp = fopen(filename, "r");
if (fp == NULL) {
printf("Failed to open file %s\n", filename);
return;
}
int i = 0;
while (fscanf(fp, "%s%s%d%s", contacts[i].name, contacts[i].department, &contacts[i].age, contacts[i].phone) != EOF) {
i++;
}
*n = i;
fclose(fp);
}
// 将联系人信息插入到二叉排序树中
void insert(Tree *root, Contact c) {
if (*root == NULL) {
*root = (Tree)malloc(sizeof(TreeNode));
(*root)->data = c;
(*root)->left = NULL;
(*root)->right = NULL;
return;
}
if (c.age <= (*root)->data.age) {
insert(&((*root)->left), c);
} else {
insert(&((*root)->right), c);
}
}
// 先序遍历二叉排序树
void preOrder(Tree root) {
if (root == NULL) {
return;
}
printf("%s %s %d %s\n", root->data.name, root->data.department, root->data.age, root->data.phone);
preOrder(root->left);
preOrder(root->right);
}
// 后序遍历二叉排序树,查找指定姓名的联系人
void postOrder(Tree root, char *name) {
if (root == NULL) {
return;
}
postOrder(root->left, name);
postOrder(root->right, name);
if (strcmp(root->data.name, name) == 0) {
printf("%s %s %d %s\n", root->data.name, root->data.department, root->data.age, root->data.phone);
}
}
// 后序遍历二叉排序树,查找指定部门的联系人
void postOrder2(Tree root, char *department) {
if (root == NULL) {
return;
}
postOrder2(root->left, department);
postOrder2(root->right, department);
if (strcmp(root->data.department, department) == 0) {
printf("%s %s %d %s\n", root->data.name, root->data.department, root->data.age, root->data.phone);
}
}
// 中序遍历二叉排序树,输出青年教师(<=35岁)的联系人信息
void inOrder(Tree root) {
if (root == NULL) {
return;
}
inOrder(root->left);
if (root->data.age <= 35) {
printf("%s %s %d %s\n", root->data.name, root->data.department, root->data.age, root->data.phone);
}
if (root->data.age <= 35) {
inOrder(root->right);
}
}
int main() {
// 从文件中读取通讯录信息
Contact contacts[100];
int n = 0;
readFromFile("contacts.txt", contacts, &n);
// 将联系人信息插入到二叉排序树中
Tree root = NULL;
for (int i = 0; i < n; i++) {
insert(&root, contacts[i]);
}
// 先序遍历二叉排序树,显示所有教师信息
printf("All teachers:\n");
preOrder(root);
// 后序遍历二叉排序树,按照姓名查询某位教师通讯信息
printf("Enter the name to search:\n");
char name[20];
scanf("%s", name);
postOrder(root, name);
// 后序遍历二叉排序树,按照部门查询该部门所有教师的通讯信息
printf("Enter the department to search:\n");
char department[20];
scanf("%s", department);
postOrder2(root, department);
// 中序遍历二叉排序树,输出青年教师(<=35岁)的联系人信息
printf("Young teachers:\n");
inOrder(root);
return 0;
}
```
阅读全文
相关推荐
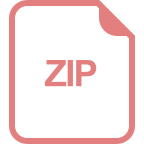
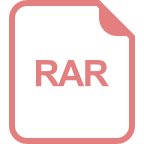










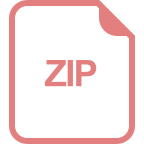
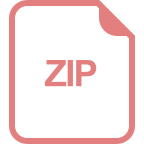
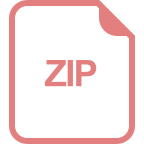
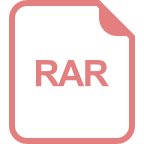
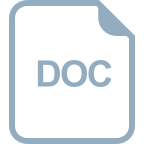
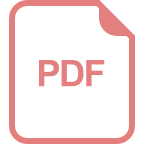