在Tekla中通过编程获得COG_X,COG_Y,COG_Z,并说明
时间: 2023-08-16 12:07:10 浏览: 203
在Tekla中,可以通过编程获取构件(Part)的重心位置(COG_X、COG_Y、COG_Z)。以下是一种可能的实现方式:
1. 获取构件的几何信息:
```csharp
Part part = ...; // 获取构件对象
Solid solid = part.GetSolid(); // 获取构件的实体几何对象
```
2. 计算几何重心:
```csharp
double volume = solid.Volume; // 获取构件体积
double cx = 0, cy = 0, cz = 0; // 初始化重心坐标
foreach (var face in solid.Faces) // 遍历构件表面
{
foreach (var loop in face.Loops) // 遍历表面环
{
foreach (var vertex in loop.Vertices) // 遍历环上的顶点
{
cx += vertex.X; // 累加 X 坐标
cy += vertex.Y; // 累加 Y 坐标
cz += vertex.Z; // 累加 Z 坐标
}
}
}
cx /= (solid.Faces.Count * 3); // 取平均值
cy /= (solid.Faces.Count * 3);
cz /= (solid.Faces.Count * 3);
cx *= volume; // 乘以体积,得到几何重心坐标
cy *= volume;
cz *= volume;
```
3. 转换坐标系:
以上计算得到的坐标是构件局部坐标系下的重心位置,需要转换为 Tekla 坐标系下的位置。
```csharp
TransformationPlane local = part.GetCoordinateSystem(); // 获取构件局部坐标系
TransformationPlane global = new TransformationPlane(); // 初始化为 Tekla 坐标系
Point point = new Point(cx, cy, cz); // 构建几何重心点对象
point.Transform(local.Transformation); // 转换为 Tekla 坐标系下的点
point.Transform(global.Inverse()); // 转换为全局坐标系下的点
double cogX = point.X; // 获取 X 坐标
double cogY = point.Y; // 获取 Y 坐标
double cogZ = point.Z; // 获取 Z 坐标
```
这样就可以得到构件的重心位置(COG_X、COG_Y、COG_Z)。需要注意的是,以上代码仅作为参考,实际应用中需要考虑更多的边界条件和异常情况。
阅读全文
相关推荐












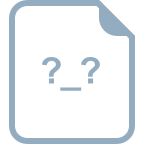