Python学校教材管理系统实现增删查改Python代码
时间: 2023-08-27 14:16:00 浏览: 96
以下是一个简单的Python学校教材管理系统的增删查改代码示例:
```python
import os
class Textbook:
def __init__(self, name, author, publisher):
self.name = name
self.author = author
self.publisher = publisher
class TextbookManager:
def __init__(self):
self.textbooks = []
def add_textbook(self, textbook):
self.textbooks.append(textbook)
def remove_textbook(self, name, author):
for textbook in self.textbooks:
if textbook.name == name and textbook.author == author:
self.textbooks.remove(textbook)
return True
return False
def search_textbook(self, name, author):
for textbook in self.textbooks:
if textbook.name == name and textbook.author == author:
return textbook
return None
def update_textbook(self, old_name, old_author, new_textbook):
for textbook in self.textbooks:
if textbook.name == old_name and textbook.author == old_author:
textbook.name = new_textbook.name
textbook.author = new_textbook.author
textbook.publisher = new_textbook.publisher
return True
return False
def save_textbooks(self, filename):
with open(filename, "w") as f:
for textbook in self.textbooks:
f.write("{},{},{}\n".format(textbook.name, textbook.author, textbook.publisher))
def load_textbooks(self, filename):
if not os.path.exists(filename):
return
with open(filename, "r") as f:
lines = f.readlines()
for line in lines:
parts = line.strip().split(",")
name = parts[0]
author = parts[1]
publisher = parts[2]
self.add_textbook(Textbook(name, author, publisher))
def main():
manager = TextbookManager()
manager.load_textbooks("textbooks.txt")
while True:
print("1. Add Textbook")
print("2. Remove Textbook")
print("3. Search Textbook")
print("4. Update Textbook")
print("5. Save Textbooks")
print("6. Exit")
choice = input("Enter your choice: ")
if choice == "1":
name = input("Enter textbook name: ")
author = input("Enter textbook author: ")
publisher = input("Enter textbook publisher: ")
manager.add_textbook(Textbook(name, author, publisher))
print("Textbook added successfully!")
elif choice == "2":
name = input("Enter textbook name: ")
author = input("Enter textbook author: ")
if manager.remove_textbook(name, author):
print("Textbook removed successfully!")
else:
print("Textbook not found!")
elif choice == "3":
name = input("Enter textbook name: ")
author = input("Enter textbook author: ")
textbook = manager.search_textbook(name, author)
if textbook:
print("Textbook found:")
print("Name:", textbook.name)
print("Author:", textbook.author)
print("Publisher:", textbook.publisher)
else:
print("Textbook not found!")
elif choice == "4":
old_name = input("Enter old textbook name: ")
old_author = input("Enter old textbook author: ")
new_name = input("Enter new textbook name: ")
new_author = input("Enter new textbook author: ")
new_publisher = input("Enter new textbook publisher: ")
new_textbook = Textbook(new_name, new_author, new_publisher)
if manager.update_textbook(old_name, old_author, new_textbook):
print("Textbook updated successfully!")
else:
print("Textbook not found!")
elif choice == "5":
manager.save_textbooks("textbooks.txt")
print("Textbooks saved successfully!")
elif choice == "6":
break
else:
print("Invalid choice!")
if __name__ == "__main__":
main()
```
上述代码定义了`Textbook`和`TextbookManager`两个类,`Textbook`类表示教材的属性,`TextbookManager`类封装了增加、删除、查询、修改等功能。在`main`函数中,我们通过循环让用户输入选择来实现不同功能的调用。同时,我们使用文件存储教材信息,可以在程序退出时保存教材信息。
阅读全文
相关推荐




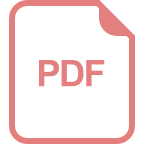
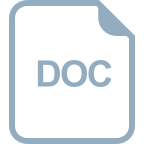
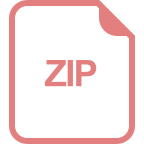
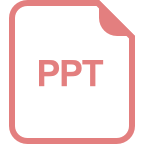
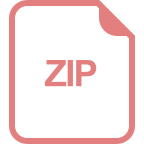
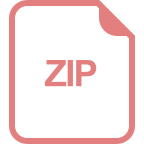
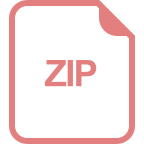
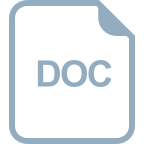