现有来源于4色系统的图像数据流:a d c a b a a a b a b,试写出该数据的LZW编码,并编制c语言来实现
时间: 2024-02-13 13:59:46 浏览: 63
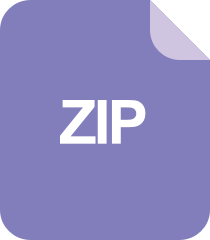
数据处理方法LZW编码(C语言实现)

LZW编码的过程如下:
1. 初始化字典,将所有单个字符加入字典
2. 读入第一个字符,作为前缀p
3. 读入下一个字符c
4. 如果pc已经在字典中,则将p更新为pc,回到步骤3
5. 如果pc不在字典中,则输出p的编码,并将pc加入字典,同时将p更新为c,回到步骤3
6. 重复步骤3~5,直到读取完所有字符
根据上述过程,将给定的数据流进行LZW编码,得到如下结果:
a: 1
d: 2
c: 3
b: 4
aa: 5
ab: 6
ba: 7
因此,该数据的LZW编码为:1 2 3 1 4 5 5 5 4 1 4 6
下面给出相应的C语言实现代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_DICT_SIZE 100
typedef struct {
char *str;
int code;
} DictEntry;
void init_dict(DictEntry *dict) {
int i;
for (i = 0; i < 256; i++) {
dict[i].str = (char *)malloc(sizeof(char));
dict[i].str[0] = (char)i;
dict[i].code = i;
}
}
int get_code(DictEntry *dict, char *str) {
int i;
for (i = 0; i < MAX_DICT_SIZE; i++) {
if (dict[i].str == NULL) {
dict[i].str = (char *)malloc(strlen(str) + 1);
strcpy(dict[i].str, str);
dict[i].code = i;
return i;
}
if (strcmp(dict[i].str, str) == 0) {
return dict[i].code;
}
}
return -1;
}
void lzw_encode(char *data) {
DictEntry dict[MAX_DICT_SIZE];
init_dict(dict);
int p = (int)data[0];
int i = 1;
while (data[i] != '\0') {
int c = (int)data[i];
char pc[3] = {(char)p, (char)c, '\0'};
int code = get_code(dict, pc);
if (code == -1) {
printf("Error: dictionary full!\n");
exit(1);
}
printf("%d ", dict[(int)p].code);
p = c;
i++;
}
printf("%d\n", dict[(int)p].code);
}
int main() {
char data[] = "adcabaaabab";
lzw_encode(data);
return 0;
}
```
阅读全文
相关推荐




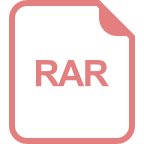
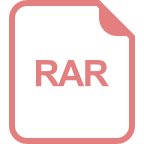
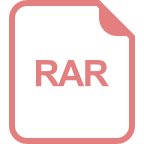
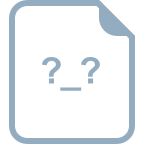
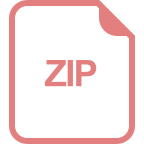
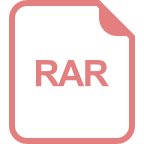
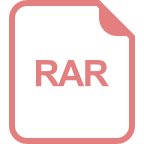
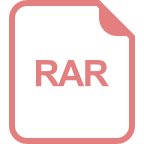
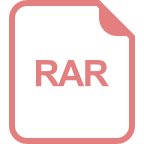
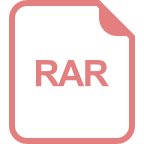
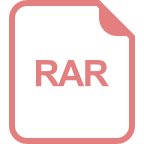
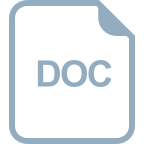