tensorflow定义多层神经元训练输入值为15,输出为1的神经网络模型,用测试数据验证模型,并保存模型代码
时间: 2023-09-13 16:05:14 浏览: 112
### 回答1:
首先需要导入tensorflow库,然后定义输入层、隐藏层和输出层的神经元个数,如输入层为15个,隐藏层为10个,输出层为1个。
```python
import tensorflow as tf
# 定义输入层、隐藏层和输出层的神经元个数
input_size = 15
hidden_size = 10
output_size = 1
```
接下来定义输入层、隐藏层和输出层的权重和偏置。
```python
# 定义输入层到隐藏层的权重和偏置
W1 = tf.Variable(tf.random.normal([input_size, hidden_size]))
b1 = tf.Variable(tf.random.normal([hidden_size]))
# 定义隐藏层到输出层的权重和偏置
W2 = tf.Variable(tf.random.normal([hidden_size, output_size]))
b2 = tf.Variable(tf.random.normal([output_size]))
```
定义输入值和预测值,并使用sigmoid激活函数进行非线性变换。
```python
# 定义输入值
x = tf.placeholder(tf.float32, [None, input_size])
# 定义隐藏层的输出值
hidden = tf.sigmoid(tf.matmul(x, W1) + b1)
# 定义预测值
y_pred = tf.sigmoid(tf.matmul(hidden, W2) + b2)
```
定义损失函数和优化器,并使用训练数据进行训练。
```python
# 定义真实值
y_true = tf.placeholder(tf.float32, [None, output_size])
# 定义损失函数
loss = tf.reduce_mean(tf.square(y_pred - y_true))
# 定义优
### 回答2:
TensorFlow是一个开源的人工智能框架,可以用于构建和训练神经网络模型。在构建多层神经网络模型时,可以通过定义多层神经元来提升模型的性能。
对于输入值为15、输出为1的神经网络模型,可以按照以下步骤进行:
1. 导入TensorFlow库
```python
import tensorflow as tf
```
2. 定义模型的输入和输出
```python
input_size = 15
output_size = 1
```
3. 定义神经网络模型的结构
```python
model = tf.keras.models.Sequential([
tf.keras.layers.Dense(32, activation='relu', input_shape=(input_size,)),
tf.keras.layers.Dense(32, activation='relu'),
tf.keras.layers.Dense(output_size, activation='linear')
])
```
这个模型包含了两个隐藏层,每个隐藏层有32个神经元。激活函数使用ReLU,最后一层使用线性激活函数。
4. 编译模型
```python
model.compile(optimizer='adam', loss='mean_squared_error')
```
使用Adam优化器和均方误差损失函数进行模型的编译。
5. 准备训练数据
```python
x_train = ... # shape为(样本数, 15)
y_train = ... # shape为(样本数, 1)
```
准备训练数据集,其中x_train为输入值,y_train为对应的输出值。
6. 训练模型
```python
model.fit(x_train, y_train, epochs=10, batch_size=32)
```
使用训练数据对模型进行训练,迭代10次,每次训练使用32个样本。
7. 准备测试数据
```python
x_test = ... # shape为(样本数, 15)
y_test = ... # shape为(样本数, 1)
```
8. 使用测试数据验证模型
```python
loss = model.evaluate(x_test, y_test)
print('测试数据的损失值:', loss)
```
使用测试数据对模型进行评估,得到损失值。
9. 保存模型代码
```python
model.save('my_model.h5')
```
将训练好的模型保存为HDF5文件,文件名为'my_model.h5'。
这样就完成了定义多层神经元训练输入值为15、输出为1的神经网络模型,并对模型进行测试和保存的过程。
阅读全文
相关推荐
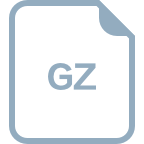





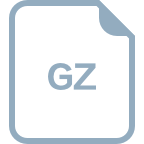










