//Copyright (c) 2020 KEYENCE CORPORATION. All rights reserved. #include <stdio.h> #include <stdlib.h> #include <string> #include <fstream> #include "CsvConverter.h" using namespace std; static int COLLECT_VALUE = 32768; static double INVALID_VALUE = -999.9999; //保存激光轮廓数据到CSV文件 //定义了一个名为CsvConverter的类,并包含了一个名为Save的函数。 //函数接受保存路径(savePath)、图像数据指针(image)、行数(lines)、列数(width)和Z轴间距(z_pitch_um)作为输入参数 long CsvConverter::Save(string savePath, unsigned short *image, int lines, int width, float z_pitch_um) { // Save the profile创建用于保存数据的文件流 ofstream stream(savePath); if (!stream) return -1; //// 定义指向图像数据的指针 unsigned short *ptr = (unsigned short*)&image[0]; //// 创建缓冲区 char buffer[20]; //遍历每一行数据 for (int i = 0; i < lines; i++) { //// 遍历每一列数据 for (int j = 0; j < width; j++) { //// 计算每个像素的实际数值 double value = *ptr == 0 ? INVALID_VALUE : (*ptr - COLLECT_VALUE) * z_pitch_um / 1000; //将实际数值转换为字符串并写入缓冲区 int length = sprintf_s(buffer, "%-.4f", value); //将数据写入文件流 stream.write((char*)buffer, length * sizeof(char)); if (j != (width - 1)) stream.write(",", sizeof(char));//// 如果不是当前行的最后一个像素,则写入逗号分隔符 ptr++;//// 指针向后移动一个位置 } stream << std::endl;//// 写入换行符 } stream.close();//// 关闭文件流 return 0;// 返回操作结果,0表示成功 } 把这个改为python
时间: 2023-12-24 21:05:58 浏览: 84
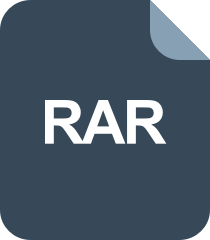
VB_Src.rar_KEYENCE LKIF.DLL_LK_keyence_keyence LKIF2.dll_keyenc

你可以将C++代码转换为等效的Python代码。以下是将给定的C++代码转换为Python的示例:
```python
COLLECT_VALUE = 32768
INVALID_VALUE = -999.9999
class CsvConverter:
@staticmethod
def save(savePath, image, lines, width, z_pitch_um):
try:
with open(savePath, 'w') as file:
for i in range(lines):
for j in range(width):
ptr = image[i * width + j]
value = INVALID_VALUE if ptr == 0 else (ptr - COLLECT_VALUE) * z_pitch_um / 1000
file.write(f"{value:.4f}")
if j != width - 1:
file.write(",")
file.write("\n")
return 0
except Exception as e:
print(f"Error: {str(e)}")
return -1
```
在Python中,你可以使用文件对象的`write()`方法将数据写入文件,使用`with`语句来自动关闭文件流。此外,Python中没有指针的概念,你可以直接通过索引访问图像数据。
请注意,这只是一个简单的示例,实际上,将复杂的C++代码转换为等效的Python代码可能需要更多的工作和适应性。
阅读全文
相关推荐
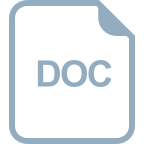
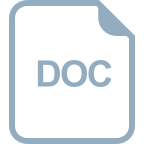
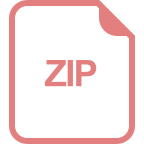
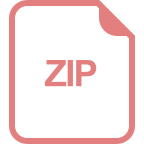
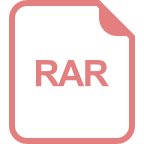
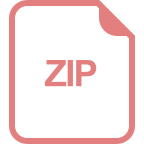
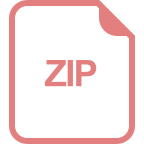
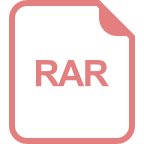
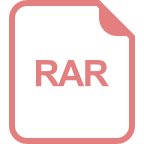
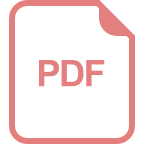
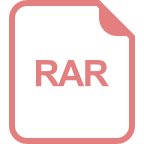
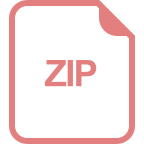
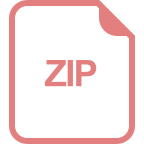
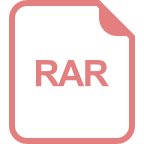
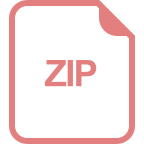

