运用结构体的方法写一个超市管理系统c++
时间: 2024-09-30 22:13:23 浏览: 27
在C++中,我们可以使用结构体(struct)来创建一个简单的超市管理系统。首先,我们需要定义一些基本的数据成员,如商品名称、价格、库存等。然后,我们可以创建一个类来管理这些商品,并提供添加、删除、查询商品等功能。下面是一个简化的示例:
```cpp
// 定义商品结构体
struct Product {
string name;
double price;
int stock;
public:
// 构造函数
Product(string n, double p, int s) : name(n), price(p), stock(s) {}
// 显示商品信息
void display() {
cout << "Name: " << name << ", Price: " << price << ", Stock: " << stock << endl;
}
};
// 超市类,包含商品列表
class Supermarket {
private:
vector<Product> products;
public:
// 添加商品
void addProduct(Product newProduct) {
products.push_back(newProduct);
}
// 删除商品
void removeProduct(const string& productName) {
for (auto it = products.begin(); it != products.end(); ++it) {
if (it->name == productName) {
products.erase(it);
break;
}
}
}
// 查询商品
bool searchProduct(const string& productName) {
for (const auto &product : products) {
if (product.name == productName) {
return true;
}
}
return false;
}
};
int main() {
Supermarket supermarket;
// 示例操作
Product apple("Apple", 3.99, 50);
supermarket.addProduct(apple);
if (supermarket.searchProduct("Apple")) {
apple.display();
} else {
cout << "Product not found." << endl;
}
return 0;
}
```
阅读全文
相关推荐
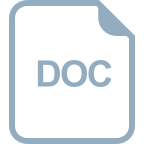
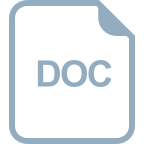
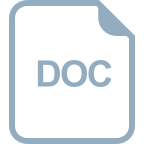
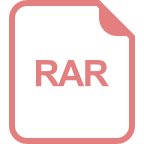
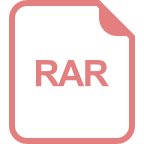
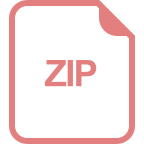
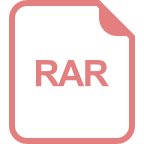
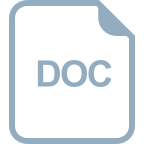
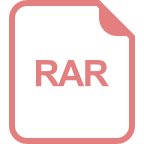
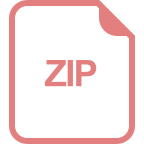
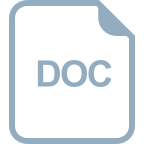
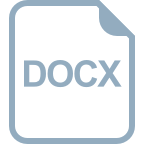
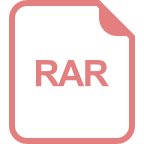
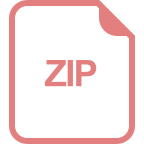
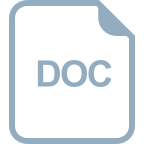
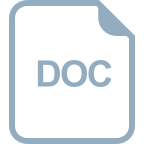
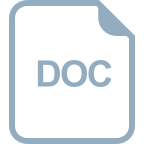
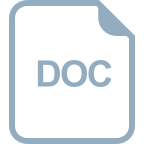
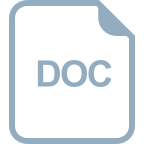