模拟退火的粒子群算法python代码
时间: 2024-10-05 15:04:27 浏览: 58
模拟退火粒子群优化(Simulated Annealing Particle Swarm Optimization, SAPSO)是一种结合了模拟退火算法和粒子群优化(PSO)策略的搜索方法。它通过模拟金属冷却过程中的相变过程,允许在局部最优解附近探索全局最优解。以下是简单的Python实现框架:
```python
import numpy as np
class SimulatedAnnealingPSO:
def __init__(self, n_particles, dim, bounds, max_iter, t_initial, t_min, alpha):
self.n_particles = n_particles
self.dim = dim
self.bounds = bounds
self.max_iter = max_iter
self.t_initial = t_initial
self.t_min = t_min
self.alpha = alpha
self.particles = np.random.uniform(bounds[0], bounds[1], (n_particles, dim))
self.velocities = np.zeros_like(self.particles)
self.best_positions = self.particles.copy()
self.global_best_position = self.particles[np.argmax(np.sum(self.particles**2, axis=1))]
def cooling_schedule(self, iteration):
return self.t_initial * np.exp(-iteration / self.max_iter)
def move_particles(self, particles, velocities, best_positions, global_best_position, temperature):
for i in range(len(particles)):
r1, r2 = np.random.rand(2)
velocities[i] = self.inertia_term(velocities[i], r1) + \
c1 * r2 * (best_positions[i] - particles[i]) + \
c2 * r2 * (global_best_position - particles[i])
if np.random.rand() < np.exp(-(np.sum((velocities[i] + particles[i]) ** 2) - np.sum(particles[i]**2)) / (2 * temperature)):
particles[i] += velocities[i]
if not self.is_valid(particles[i]):
particles[i] = self.resample(particles[i])
def inertia_term(self, velocity, r):
return velocity * np.where(r < 0.5, 2*r, 2*(1-r))
# 辅助函数:检查新位置是否有效,并进行随机重采样
def is_valid(self, position):
return all(position >= self.bounds[0]) and all(position <= self.bounds[1])
def resample(self, position):
valid_pos = np.clip(position, self.bounds[0], self.bounds[1])
return valid_pos
def optimize(self):
for iteration in range(self.max_iter):
temperature = self.cooling_schedule(iteration)
self.move_particles(self.particles, self.velocities, self.best_positions, self.global_best_position, temperature)
self.update_best_positions()
return self.global_best_position
# 使用示例:
n_particles = 50
dim = 10
bounds = (-5, 5)
max_iter = 1000
t_initial = 1
t_min = 0.01
alpha = 0.9
algorithm = SimulatedAnnealingPSO(n_particles, dim, bounds, max_iter, t_initial, t_min, alpha)
result = algorithm.optimize()
print("全局最佳解:", result)
```
这个代码创建了一个`SimulatedAnnealingPSO`类,包含了粒子初始化、温度调整、移动粒子以及更新最佳位置等核心步骤。你可以根据需要调整参数进行实际优化。
阅读全文
相关推荐
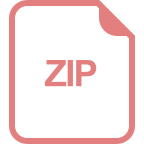
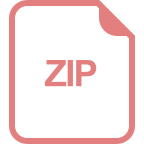
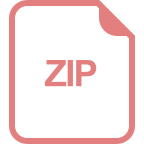


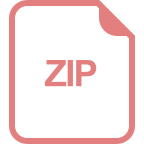
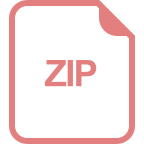
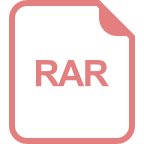
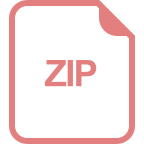
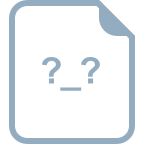
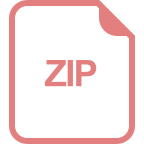
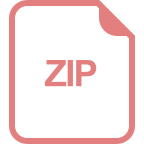
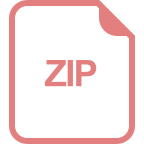
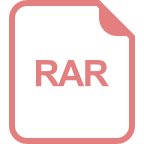



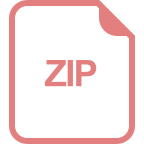
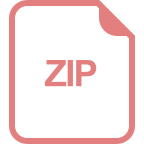