python编写代码,实现将用户输入的十进制整数转换为指定进制(二进制、八进制、十六进制)的功能。要求分别用到%格式化、format()方法、f-string三种方式进行输出。
时间: 2024-09-28 19:05:15 浏览: 8
在Python中,我们可以利用取模运算符 `%`、`format()` 函数以及 f-string 来实现这个功能。以下是三种方式的具体示例:
1. 使用 `%` 格式化:
```python
def dec_to_other(base):
number = int(input("请输入一个十进制整数: "))
binary = bin(number)[2:] if base == 2 else "Invalid base"
octal = oct(number)[2:] if base == 8 else "Invalid base"
hex_num = hex(number)[2:].upper() if base == 16 else "Invalid base"
print(f"二进制: {binary}")
print(f"八进制: {octal}")
print(f"十六进制: {hex_num}")
dec_to_other(2)
```
2. 使用 `format()` 方法:
```python
def dec_to_other_format(base):
number = int(input("请输入一个十进制整数: "))
binary = "{0:b}".format(number) if base == 2 else "Invalid base"
octal = "{0:o}".format(number) if base == 8 else "Invalid base"
hex_num = "{0:x}".format(number).upper() if base == 16 else "Invalid base"
print("二进制:", binary)
print("八进制:", octal)
print("十六进制:", hex_num)
dec_to_other_format(2)
```
3. 使用 f-string:
```python
def dec_to_other_fstring(base):
number = int(input("请输入一个十进制整数: "))
binary = f"{number:b}" if base == 2 else "Invalid base"
octal = f"{number:o}" if base == 8 else "Invalid base"
hex_num = f"{number:X}".upper() if base == 16 else "Invalid base"
print(f"二进制: {binary}")
print(f"八进制: {octal}")
print(f"十六进制: {hex_num}")
dec_to_other_fstring(2)
```
以上三种方式都可以实现将十进制整数转换为其他进制,当基础不是2、8或16时会显示 "Invalid base"。如果你运行上述任一函数,并输入一个十进制整数,它将会按照指定的进制进行转换并打印结果。
相关推荐
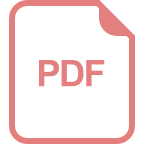
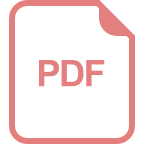
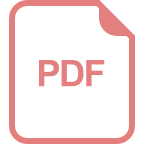
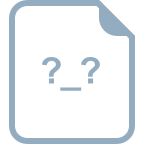
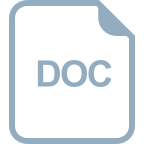












