mapreduce代码
时间: 2023-07-27 09:08:15 浏览: 81
以下是一个简单的 MapReduce 代码示例,用于计算一个文本文件中每个单词的出现次数:
```python
from collections import Counter
from multiprocessing import Pool
def map_function(line):
words = line.strip().split()
return Counter(words)
def reduce_function(counters):
return sum(counters, Counter())
def mapreduce(file_path):
with open(file_path, 'r') as file:
lines = file.readlines()
with Pool() as pool:
mapped_counts = pool.map(map_function, lines)
reduced_counts = reduce_function(mapped_counts)
return reduced_counts
if __name__ == '__main__':
file_path = 'path_to_your_text_file.txt'
word_counts = mapreduce(file_path)
print(word_counts)
```
这段代码使用了 Python 的 `multiprocessing.Pool` 来并行执行 Map 阶段的任务。首先,`map_function` 函数将每行文本分割成单词,并返回一个 Counter 对象,其中包含每个单词的出现次数。然后,`reduce_function` 函数对所有 Counter 对象进行求和,得到最终的单词计数结果。在主函数中,我们使用 `Pool.map` 方法并行执行 Map 阶段的任务,并将结果传递给 Reduce 阶段的函数进行归约。最后,打印出每个单词及其出现次数的字典。
请注意,这只是一个简单的示例代码,实际的 MapReduce 实现可能更加复杂,具体取决于任务的要求和所使用的编程语言。
相关推荐
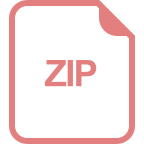













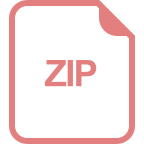