根据mac地址获取ip
时间: 2024-06-18 22:01:02 浏览: 195
在C语言中,直接根据MAC地址获取IP地址通常涉及到网络编程和可能的外部库,因为这个过程需要查询网络设备的ARP缓存或使用更复杂的网络接口API。标准C库并不直接提供这样的功能,通常我们会用到一些系统调用或者第三方库,如`libpcap`或`getifaddrs`等。
以下是一个简化的示例,使用`getifaddrs`函数从系统获取网络接口信息,然后遍历查找指定MAC地址对应的IP地址。请注意,这只是一个基础示例,实际应用可能需要处理更多的错误情况和边界条件。
```c
#include <stdio.h>
#include <net/if.h>
#include <linux/if_ether.h>
void print_ip_address(const struct ifaddrs *ifa, char *mac) {
while (ifa) {
const struct sockaddr_dl *sdl = (struct sockaddr_dl *) ifa->ifa_addr;
char mac_addr[18] = {0};
memcpy(mac_addr, LLADDR(sdl), ETH_ALEN);
mac_addr[ETH_ALEN] = '\0';
if (!memcmp(mac_addr, mac, ETH_ALEN)) {
const char *addr = inet_ntoa(((struct sockaddr_in *)&ifa->ifa_addr)->sin_addr);
printf("MAC %s has IP address: %s\n", mac_addr, addr);
break;
}
ifa = ifa->ifa_next;
}
}
int main() {
// 获取所有网络接口信息
struct ifaddrs *ifap, *ifa;
if (getifaddrs(&ifap) == -1) {
perror("Error getting interface addresses");
return 1;
}
// 打印每个接口的MAC和IP地址
print_ip_address(ifap, "your-mac-address-here");
// 清理内存并释放资源
freeifaddrs(ifap);
return 0;
}
```
在这个例子中,你需要替换`"your-mac-address-here"`为你要查找的实际MAC地址。请注意,这个程序只能在支持`getifaddrs`的系统上运行,而且不是所有系统都支持直接获取MAC到IP的映射关系,有些情况下需要查询ARP缓存或者网络配置。
阅读全文
相关推荐
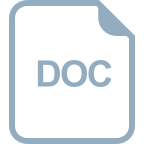
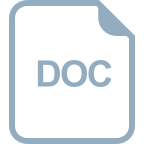
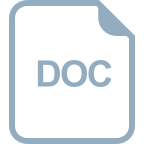



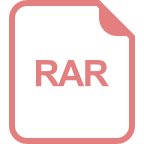
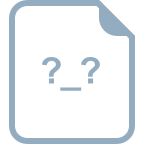
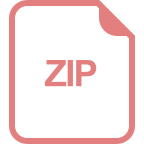
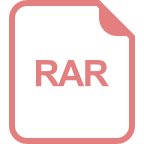
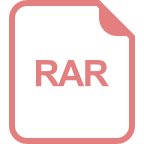
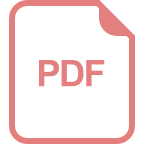
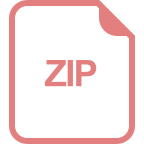
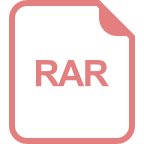

