数据结构顺序表采用顺序存储的代码
时间: 2024-10-13 07:17:17 浏览: 1
顺序表是一种常见的数据结构,它通过连续的内存空间存储元素,通常数组是最常用的实现方式。以下是使用C++实现基本顺序表的基本代码:
```cpp
#include <iostream>
// 定义顺序表的节点
template <typename T>
struct ListNode {
T data;
ListNode* next; // 指向下一个节点的指针
};
// 定义顺序表类
template <typename T>
class SeqList {
private:
ListNode<T>* head; // 表头节点
int size; // 当前元素的数量
public:
SeqList() : head(nullptr), size(0) {}
// 插入元素到列表尾部
void insert(T value) {
ListNode<T>* newNode = new ListNode<T>{value};
if (!head) {
head = newNode;
} else {
ListNode<T>* current = head;
while (current->next != nullptr) {
current = current->next;
}
current->next = newNode;
}
size++;
}
// 删除指定值的第一个匹配项
void remove(const T& value) {
if (!head || head->data == value) {
delete head;
head = head->next;
if (head)
size--;
return;
}
ListNode<T>* current = head;
while (current->next && current->next->data != value) {
current = current->next;
}
if (current->next) {
delete current->next;
current->next = current->next->next;
size--;
}
}
// 显示顺序表内容
void display() const {
ListNode<T>* temp = head;
while (temp) {
std::cout << temp->data << " ";
temp = temp->next;
}
std::cout << "\n";
}
// 获取顺序表大小
int getSize() const { return size; }
};
int main() {
SeqList<int> list;
list.insert(1);
list.insert(2);
list.insert(3);
list.display(); // 输出: 1 2 3
list.remove(2);
list.display(); // 输出: 1 3
return 0;
}
```
在这个例子中,我们创建了一个`ListNode`模板类作为顺序表的内部节点,以及一个`SeqList`模板类来管理这些节点。主要操作包括插入、删除和显示列表内容。
相关推荐
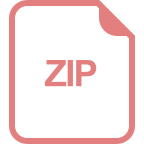
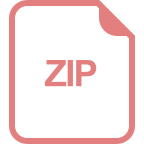
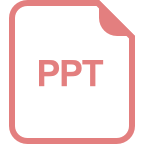














