数据结构中顺序表的取值代码
时间: 2024-09-24 22:24:54 浏览: 36
顺序表是一种线性的数据结构,它通过连续的内存空间存储元素,每个元素都有一个固定的索引。在大多数编程语言中,访问顺序表的某个元素通常涉及以下步骤:
```python
# Python 示例
class SequentialList:
def __init__(self):
self.data = []
# 添加元素到列表尾部
def append(self, value):
self.data.append(value)
# 取值操作,假设我们有一个整数列表
def get_value(self, index):
if 0 <= index < len(self.data): # 检查索引是否越界
return self.data[index] # 如果在范围内,返回对应位置的值
else:
return None # 否则返回None表示索引错误
# 使用示例
list = SequentialList()
list.append(1)
list.append(2)
print(list.get_value(0)) # 输出 1
```
相关问题
数据结构顺序表实验一c++ 1.线性表顺序存储结构下基本操作的实现(初始化、建表、取值、查找、插入、删除、两个非递减有序链表的归并等)。 2.线性表链式存储结构下基本操作的实现(初始化、建表、取值、查找、插入、删除、两个非递减有序链表的归并等)。生成源代码
在C++中,实现数据结构的顺序表和链表的基本操作可以分为两部分:
**1. 线性表顺序存储结构(数组实现):**
```cpp
#include <iostream>
// 定义顺序表节点和列表
template <typename T>
class ArrayList {
private:
T* data; // 存储元素的数组
size_t size; // 当前元素数量
static const size_t CAPACITY = 10; // 初始容量
public:
ArrayList() : data(new T[CAPACITY]), size(0) {}
~ArrayList() { delete[] data; }
void initialize() {
for (size_t i = 0; i < CAPACITY; ++i)
data[i] = T(); // 初始化所有元素
}
// 其他基本操作如 insert, find, remove, 同理
// 示例:
void insert(int index, const T& value) {
if (index < size) {
memmove(data + index + 1, data + index, sizeof(T) * (size - index));
}
data[index] = value;
size++;
}
// 查找和删除操作同样需要考虑边界条件和索引有效性
};
// 非递减有序链表的归并示例:
template <typename Compare>
void merge(ArrayList<int>& listA, ArrayList<int>& listB, Compare compare) {
// 实现合并算法...
}
```
**2. 线性表链式存储结构(动态链接列表):**
```cpp
template <typename T>
class LinkedList {
private:
struct Node {
T data;
Node* next;
};
Node* head;
Node* tail;
public:
LinkedList() : head(nullptr), tail(nullptr) {}
void initialize() {
head = nullptr;
tail = nullptr;
}
// 插入、查找、删除等操作分别通过头结点、指针遍历等方式实现
// 例如插入操作:
void insert(const T& value) {
Node* newNode = new Node{value, nullptr};
if (!head) {
head = newNode;
tail = newNode;
} else {
tail->next = newNode;
tail = newNode;
}
}
// 归并链表操作也需处理特殊情况,比如合并后的节点排序
}
// 归并链表示例:
template <typename Compare>
void mergeLinkedLists(LinkedList<int>& listA, LinkedList<int>& listB, Compare compare) {
// 实现归并链表算法...
}
```
以上代码仅为基础框架,实际操作中还需要添加错误处理、边界检查以及详细的实现细节。对于链表的归并,通常会采用迭代或递归的方式来合并两个有序链表。
**相关问题--:**
1. 在顺序表和链表中,插入操作的时间复杂度有何区别?
2. 如何在链表中高效地搜索某个特定元素?
3. 对于大型数据集,你会倾向于选择哪种存储结构,并解释原因?
c语言代码请定义一个整形数组,例如:ElenmTvpe datar97 = 11 .-22.33-3-8821.77.091将其插入顺序表,并在表中完成顺序表的基本操作(删除、增加、查找、取值等)。
在C语言中,首先你需要创建一个结构体来表示元素,包括整数数据和其他可能的数据类型。然后定义一个顺序表(动态数组)来存储这些元素。以下是创建一个简单的顺序表以及实现基本操作的示例:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义一个结构体来包含整数和浮点数
typedef struct {
int integer;
float float_value;
} Element;
// 动态数组结构,包含元素和大小
typedef struct {
Element* data; // 指向元素的指针
int size; // 当前元素的数量
int capacity; // 数组的最大容量
} List;
// 初始化列表
void init_list(List* list) {
list->data = (Element*)malloc(sizeof(Element));
list->size = 0;
list->capacity = 5; // 初始容量设为5
}
// 插入元素
void insert(List* list, int index, int value, float flt_val) {
if (index > list->size || index < 0) {
printf("Invalid index.\n");
return;
}
if (list->size == list->capacity) {
// 扩容数组
list->capacity *= 2;
Element* newData = realloc(list->data, sizeof(Element) * list->capacity);
if (!newData) {
printf("Memory allocation failed.\n");
return;
}
list->data = newData;
}
list->data[index].integer = value;
list->data[index].float_value = flt_val;
list->size++;
}
// 删除元素
void delete(List* list, int index) {
if (index >= list->size) {
printf("Index out of bounds.\n");
return;
}
memmove(&list->data[index], &list->data[index + 1], (list->size - index - 1) * sizeof(Element));
list->size--;
}
// 查找元素
int find(List* list, int value) {
for (int i = 0; i < list->size; i++) {
if (list->data[i].integer == value)
return i;
}
return -1; // 如果未找到,返回-1
}
// 取值
void get_value(List* list, int index, int* int_val, float* flt_val) {
if (index >= list->size) {
printf("Index out of bounds.\n");
return;
}
*int_val = list->data[index].integer;
*flt_val = list->data[index].float_value;
}
int main() {
List exampleList;
init_list(&exampleList);
// 插入元素
insert(&exampleList, 0, 11, -22.33);
insert(&exampleList, 2, 3, 88.21); // 注意索引从0开始
// 其他操作...
int val_to_find = 11;
int found_index = find(&exampleList, val_to_find);
if (found_index != -1) {
printf("Found %d at index %d\n", val_to_find, found_index);
}
return 0;
}
```
阅读全文
相关推荐
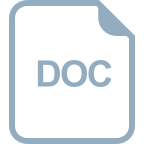
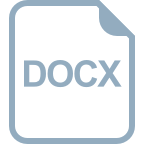
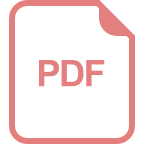
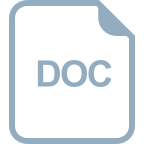
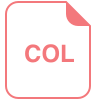










