顺序表的基本操作初始化,赋值,遍历,取值,求表长,插入,查找,删除,退出示例代码
时间: 2024-09-22 20:06:33 浏览: 34
顺序表是一种简单的线性数据结构,通常采用数组的形式存储元素。以下是顺序表的一些基本操作及其示例代码:
1. **初始化**:
- C++ 示例:
```cpp
int List[10]; // 创建一个长度为10的整数顺序表
```
2. **赋值**:
- C++ 示例: 给第i个位置赋值
```cpp
List[i] = value; // i从0开始
```
3. **遍历**:
- C++ 示例 (使用for循环):
```cpp
for(int i=0; i<10; i++) {
cout << List[i] << " ";
}
```
- Python 示例:
```python
for item in List:
print(item)
```
4. **取值**:
- C++ 示例:
```cpp
int val = List[3]; // 取第四个元素
```
5. **求表长**:
- C++ 示例:
```cpp
int length = sizeof(List) / sizeof(List[0]); // 表长等于数组长度
```
- Python 示例:
```python
length = len(List)
```
6. **插入**:
- C++ 示例 (插入到特定索引,假设列表有扩容功能):
```cpp
List insertIndex = 2;
List[insertIndex] = newValue;
```
- Python 示例:
```python
List.insert(insertIndex, newValue)
```
7. **查找**:
- C++ 示例:
```cpp
int index = find(List, target); // 查找target的位置,返回其下标,未找到则返回-1
```
- Python 示例:
```python
index = List.index(target) if target in List else None
```
8. **删除**:
- C++ 示例 (删除指定索引处的元素,假设列表动态管理空间):
```cpp
delete List[delIndex];
```
- Python 示例:
```python
del List[delIndex]
```
9. **退出/关闭**:
- 顺序表不需要显式退出,因为它们是内存分配的一部分,直到程序结束才会释放。
相关推荐
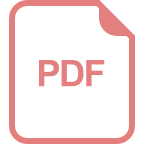
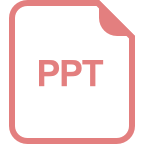
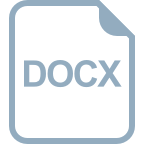

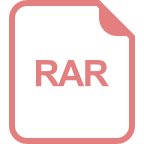
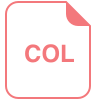
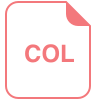
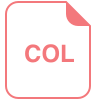
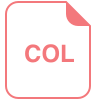

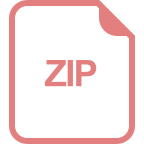
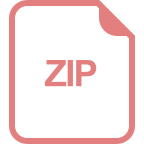
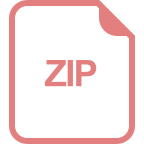
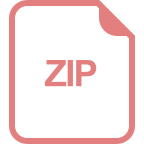
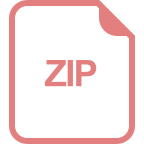
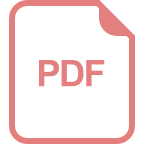