写一个银行取款、存款、转账的前端页面
时间: 2023-07-10 22:34:59 浏览: 86
好的,以下是一个简单的银行取款、存款、转账的前端页面的代码示例,使用了HTML、CSS和JavaScript:
```html
<!DOCTYPE html>
<html>
<head>
<title>Bank Account</title>
<style>
body {
font-family: Arial, sans-serif;
}
h1 {
text-align: center;
}
fieldset {
margin: 2em auto;
padding: 1em;
width: 50%;
}
label {
display: block;
margin-bottom: 0.5em;
}
input[type="number"] {
width: 100%;
padding: 0.5em;
border-radius: 5px;
border: 1px solid gray;
}
button {
display: block;
margin-top: 1em;
padding: 0.5em;
border-radius: 5px;
border: none;
background-color: #4CAF50;
color: white;
font-size: 1em;
cursor: pointer;
}
button:hover {
background-color: #3e8e41;
}
</style>
</head>
<body>
<h1>Bank Account</h1>
<fieldset>
<legend>Deposit</legend>
<label for="deposit-amount">Amount:</label>
<input type="number" id="deposit-amount" name="deposit-amount">
<button id="deposit-button">Deposit</button>
</fieldset>
<fieldset>
<legend>Withdraw</legend>
<label for="withdraw-amount">Amount:</label>
<input type="number" id="withdraw-amount" name="withdraw-amount">
<button id="withdraw-button">Withdraw</button>
</fieldset>
<fieldset>
<legend>Transfer</legend>
<label for="transfer-amount">Amount:</label>
<input type="number" id="transfer-amount" name="transfer-amount">
<label for="transfer-account">Account:</label>
<input type="text" id="transfer-account" name="transfer-account">
<button id="transfer-button">Transfer</button>
</fieldset>
<script>
const depositButton = document.getElementById('deposit-button');
const withdrawButton = document.getElementById('withdraw-button');
const transferButton = document.getElementById('transfer-button');
depositButton.addEventListener('click', () => {
const amount = parseFloat(document.getElementById('deposit-amount').value);
if (isNaN(amount) || amount < 0) {
alert('Deposit amount must be a positive number.');
} else {
// Call backend API to deposit amount
alert(`Deposit successful. Current balance is $${amount}.`);
}
});
withdrawButton.addEventListener('click', () => {
const amount = parseFloat(document.getElementById('withdraw-amount').value);
if (isNaN(amount) || amount < 0) {
alert('Withdrawal amount must be a positive number.');
} else {
// Call backend API to withdraw amount
alert(`Withdrawal successful. Current balance is $${amount}.`);
}
});
transferButton.addEventListener('click', () => {
const amount = parseFloat(document.getElementById('transfer-amount').value);
const account = document.getElementById('transfer-account').value;
if (isNaN(amount) || amount < 0) {
alert('Transfer amount must be a positive number.');
} else if (account === '') {
alert('Transfer account must not be empty.');
} else {
// Call backend API to transfer amount to account
alert(`Transfer successful. $${amount} transferred to account ${account}.`);
}
});
</script>
</body>
</html>
```
这个页面包含三个fieldset,分别是存款、取款和转账。每个fieldset包含一些标签和输入框,以及一个按钮,用于提交操作。JavaScript代码使用addEventListener()将每个按钮与相应的操作相关联,检查输入的值是否合法(必须是正数),然后向后端API发送请求,完成相应的操作。在这个简单的示例中,我们使用JavaScript中的alert()方法显示成功或失败的消息,但在实际应用中,我们可能会将消息显示在页面上,或者使用一些其他的提示方式。
相关推荐
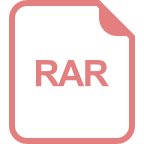










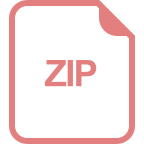
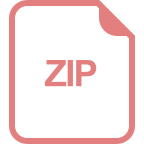
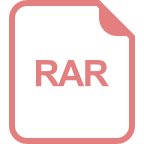