用c语言编程文曲星游戏
时间: 2024-03-13 18:17:45 浏览: 144
文曲星游戏是一款文字冒险游戏,可以用C语言编写。下面是一个简单的代码示例:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_ROOMS 10
#define MAX_ITEMS 5
typedef struct {
char name[50];
char description[100];
int exits[4];
int num_items;
char items[MAX_ITEMS][50];
} Room;
typedef struct {
char name[50];
char description[100];
} Item;
Room rooms[MAX_ROOMS];
Item items[MAX_ITEMS];
int current_room = 0;
int inventory[MAX_ITEMS];
int num_inventory = 0;
void init_rooms() {
strcpy(rooms[0].name, "Entrance");
strcpy(rooms[0].description, "You are standing in front of a large entrance.");
rooms[0].exits[0] = -1;
rooms[0].exits[1] = -1;
rooms[0].exits[2] = 1;
rooms[0].exits[3] = -1;
rooms[0].num_items = 0;
strcpy(rooms[1].name, "Library");
strcpy(rooms[1].description, "You are in a large library filled with books.");
rooms[1].exits[0] = -1;
rooms[1].exits[1] = -1;
rooms[1].exits[2] = -1;
rooms[1].exits[3] = 0;
rooms[1].num_items = 1;
strcpy(rooms[1].items[0], "book");
// add more rooms here
}
void init_items() {
strcpy(items[0].name, "book");
strcpy(items[0].description, "A thick book with a leather cover.");
}
void print_room() {
printf("%s\n", rooms[current_room].name);
printf("%s\n", rooms[current_room].description);
printf("Exits: ");
if (rooms[current_room].exits[0] != -1) {
printf("north ");
}
if (rooms[current_room].exits[1] != -1) {
printf("east ");
}
if (rooms[current_room].exits[2] != -1) {
printf("south ");
}
if (rooms[current_room].exits[3] != -1) {
printf("west ");
}
printf("\n");
if (rooms[current_room].num_items > 0) {
printf("Items: ");
for (int i = 0; i < rooms[current_room].num_items; i++) {
printf("%s ", rooms[current_room].items[i]);
}
printf("\n");
}
}
void print_inventory() {
if (num_inventory == 0) {
printf("Your inventory is empty.\n");
} else {
printf("Your inventory:\n");
for (int i = 0; i < num_inventory; i++) {
printf("%s\n", items[inventory[i]].name);
}
}
}
int main() {
init_rooms();
init_items();
char input[100];
while (1) {
print_room();
printf("> ");
fgets(input, 100, stdin);
input[strlen(input)-1] = '\0';
if (strcmp(input, "north") == 0) {
if (rooms[current_room].exits[0] != -1) {
current_room = rooms[current_room].exits[0];
} else {
printf("You cannot go north.\n");
}
} else if (strcmp(input, "east") == 0) {
if (rooms[current_room].exits[1] != -1) {
current_room = rooms[current_room].exits[1];
} else {
printf("You cannot go east.\n");
}
} else if (strcmp(input, "south") == 0) {
if (rooms[current_room].exits[2] != -1) {
current_room = rooms[current_room].exits[2];
} else {
printf("You cannot go south.\n");
}
} else if (strcmp(input, "west") == 0) {
if (rooms[current_room].exits[3] != -1) {
current_room = rooms[current_room].exits[3];
} else {
printf("You cannot go west.\n");
}
} else if (strcmp(input, "take") == 0) {
char item_name[50];
printf("What do you want to take? ");
fgets(item_name, 50, stdin);
item_name[strlen(item_name)-1] = '\0';
for (int i = 0; i < rooms[current_room].num_items; i++) {
if (strcmp(item_name, rooms[current_room].items[i]) == 0) {
if (num_inventory == MAX_ITEMS) {
printf("Your inventory is full.\n");
} else {
inventory[num_inventory] = i;
num_inventory++;
printf("You take the %s.\n", item_name);
}
break;
}
}
} else if (strcmp(input, "inventory") == 0) {
print_inventory();
} else if (strcmp(input, "quit") == 0) {
break;
} else {
printf("Invalid command.\n");
}
}
return 0;
}
```
这个代码实现了一个简单的文曲星游戏,包括了几个房间、物品、移动、捡起物品等基本功能。你可以根据需要修改和扩展。
阅读全文
相关推荐
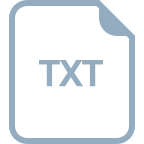
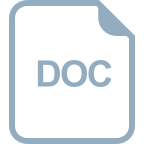
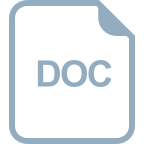
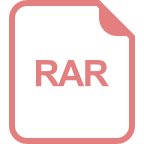
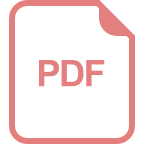
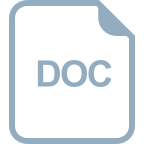
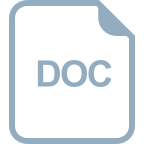
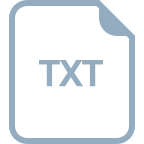
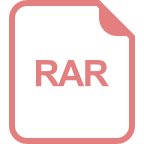
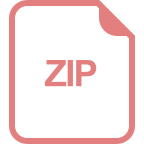
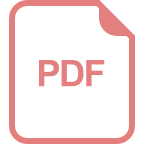
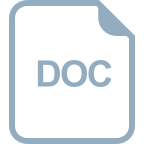
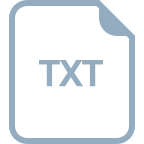
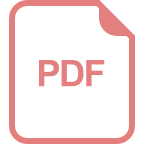