用c++写出补充后问题
时间: 2024-10-04 14:02:52 浏览: 33
在C++中设计这样一个游戏,我们需要首先创建一些数据结构来存储任务信息(例如任务ID、值等),然后定义两个玩家的类(如Player_Ayu和Player_TaoTao)。下面是一个简化的示例:
```cpp
#include <iostream>
#include <vector>
// 定义任务类
class Task {
public:
int id;
int value;
// 构造函数
Task(int id, int value) : id(id), value(value) {}
};
// 玩家类
class Player {
protected:
std::vector<Task> tasks;
int currentScore;
public:
void receiveTask(Task task) { tasks.push_back(task); }
int takeTask() {
if (tasks.empty()) return -1; // 如果无任务可选,则返回-1
Task chosenTask = tasks.back(); // 最后一个任务
tasks.pop_back();
currentScore += chosenTask.value;
return chosenTask.id; // 返回选取的任务id
}
int getCurrentScore() const { return currentScore; }
};
int main() {
Player_Ayu ayu;
Player_TaoTao taoTao;
// 初始化任务
for (int i = 0; i < numTasks; ++i) {
Task task(i, randomTaskValue());
ayu.receiveTask(task);
}
while (!tasks.empty()) {
// 阿鱼先行动
int ayuChoice = ayu.takeTask();
// 涛涛接着行动
int taoTaoChoice = ayuChoice == -1 ? ayu.takeTask() : ayuChoice;
// 更新双方得分并处理其他逻辑
// 打印当前得分
std::cout << "Ayu: " << ayu.getCurrentScore() << ", TaoTao: " << taoTao.getCurrentScore() << "\n";
}
return 0;
}
```
在这个例子中,我们假设`numTasks`是预设的任务总数,`randomTaskValue()`是一个生成随机任务价值的函数。实际游戏中还需要添加更详细的交互逻辑和状态跟踪。
阅读全文
相关推荐




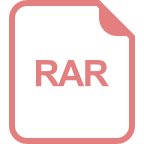













