用C++写出上面的代码
时间: 2024-09-22 18:05:16 浏览: 40
在C++中,由于标准库已经提供了一些大整数支持,比如`std::BigInt`或者`<boost/multiprecision/cpp_int>`,我们可以使用这些库来简化大整数的操作。如果没有库可用,我们可以自定义一个简单的字符串大整数类实现上述功能。下面是一个简化的例子,仅展示核心部分的代码:
```cpp
#include <iostream>
#include <string>
class BigInt {
public:
BigInt(const std::string &s = "0") : value(s) {}
// 加法
BigInt operator+(const BigInt &other) const {
return BigInt(add(value, other.value));
}
// 减法
BigInt operator-(const BigInt &other) const {
return BigInt(subtract(value, other.value));
}
// 乘法
BigInt operator*(const BigInt &other) const {
return BigInt(multiply(value, other.value));
}
// 初始版本的除法,这里省略了除法和取余的具体实现
// BigInt operator/(const BigInt &other) const;
private:
std::string value;
static std::string add(const std::string &a, const std::string &b) {
// 实现字符串加法...
}
static std::string subtract(const std::string &a, const std::string &b) {
// 实现字符串减法...
}
static std::string multiply(const std::string &a, const std::string &b) {
// 实现字符串乘法...
}
};
// 示例
int main() {
BigInt a("1234567890");
BigInt b("9876543210");
BigInt sum = a + b;
BigInt difference = a - b;
BigInt product = a * b; // 这里假设乘法已实现
// 输出结果
std::cout << "Sum: " << sum.value << "\n";
std::cout << "Difference: " << difference.value << "\n";
std::cout << "Product: " << product.value << "\n";
return 0;
}
```
请注意,这里的代码仅作演示,实际应用中你需要补充完整的字符串加减乘除函数实现,以及处理溢出和边界条件的细节。
阅读全文
相关推荐
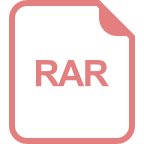
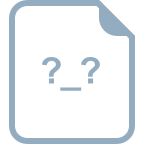
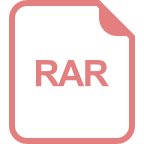















