void Load() { ifstream fin(File); string put,title,author,publisher; int page,stock,num; double price; while(fin>>put>>title>>author>>publisher>>page>>stock>>price>>num) { if(put=="入库") { Book bookk(title,author,publisher,page,stock,price); book.push_back(bookk); } else { for(int i=0;i<book.size();i++) { if(book[i].get_title()==title) { book[i].set_stock(book[i].get_stock()-num); break; } } } } fin.close(); } };
时间: 2024-02-11 17:30:33 浏览: 16
这段代码看起来是一个C++类的成员函数,函数名为Load,不接受任何参数。这个函数的功能是从文件中读取书籍信息并存储到book这个vector容器中。函数中首先创建一个ifstream对象fin,打开文件并读取文件中的信息。每行信息包括put、title、author、publisher、page、stock、price和num,表示书籍的入库或出库记录。如果put的值是"入库",则创建一个Book对象并将其添加到book容器中;如果put的值是"出库",则遍历book容器,找到对应的书籍并更新其库存量。函数执行完毕后,关闭文件。
相关问题
class Book {//书籍类 private: string name; string author; string publisher; int pages; Time latestTime; int stock; double price; 能够用list容器写该数据的文件的存储和读取。
可以使用STL中的list容器对该数据进行存储和读取操作。需要注意的是,list容器中存储的是Book对象,因此需要重载Book类的输入输出运算符。以下是一个示例代码:
```c++
#include <iostream>
#include <list>
#include <fstream>
using namespace std;
class Time {//时间类
//...
};
class Book {//书籍类
private:
string name;
string author;
string publisher;
int pages;
Time latestTime;
int stock;
double price;
public:
//重载输入输出运算符
friend ostream& operator<<(ostream& os, const Book& b) {
os << b.name << " " << b.author << " " << b.publisher << " "
<< b.pages << " " << b.latestTime << " " << b.stock << " " << b.price << endl;
return os;
}
friend istream& operator>>(istream& is, Book& b) {
is >> b.name >> b.author >> b.publisher >> b.pages
>> b.latestTime >> b.stock >> b.price;
return is;
}
};
int main() {
list<Book> books;
//写入文件
ofstream out("books.txt");
if (!out) {
cerr << "Cannot open file" << endl;
return 1;
}
for (const auto& book : books) {
out << book;
}
out.close();
//从文件中读取
ifstream in("books.txt");
if (!in) {
cerr << "Cannot open file" << endl;
return 1;
}
Book book;
while (in >> book) {
books.push_back(book);
}
in.close();
//...
return 0;
}
```
在该示例中,使用了STL中的list容器对Book对象进行存储,并通过重载输入输出运算符实现了将Book对象写入文件和从文件中读取Book对象的操作。
class BookRecord{//入库记录类 public: string name; string author; string publisher; int pages; Time latestTime; double price; int account; public: BookRecord(string n, string a, string p, int pg,Time lt , int acc,double pr): name(n), author(a), publisher(p), pages(pg), latestTime(lt),account(acc),price(pr) {} void loadData() {//读取数据:图书 ifstream inFile("data.txt"); string line; while (getline(inFile, line)) { string name, author, publisher; int pages, stock; double price; int year, month, day; sscanf(line.c_str(), "%[^,],%[^,],%[^,],%d,%d-%d-%d,%d,%lf", &name[0], &author[0], &publisher[0], &pages, &year, &month, &day, &stock, &price); Time latestTime(year, month, day); Book b(name, author, publisher, pages, latestTime, stock, price); books.push_back(b); } inFile.close(); } 数据怎么读取不成功怎么修改?
如果你在读取数据时遇到了问题,可以尝试一些调试方法来找出具体问题所在:
1. 确认文件路径:首先要确保文件路径正确,否则无法读取到数据。
2. 输出调试信息:在读取数据时,可以在每一行代码后面输出一些调试信息,比如输出读取的行数据看是否正确,或者输出变量值看是否符合预期等。
3. 检查数据格式:在读取每一行数据时,需要确保字符串格式和数据类型匹配,如果不匹配则会读取失败。可以使用一些调试工具或者输出数据类型来检查数据格式是否正确。
另外,你的代码中使用了 `sscanf` 函数来解析每一行数据,这个函数的使用需要非常谨慎,容易出现错误。你可以使用更加安全的 `std::stringstream` 来解析数据,具体实现可以参考以下代码:
```
void loadData() {
ifstream inFile("data.txt");
if (!inFile.is_open()) {
cout << "无法打开文件!\n";
return;
}
string line;
while (getline(inFile, line)) {
stringstream ss(line); // 将字符串转换为 stringstream 对象
string name, author, publisher;
int pages, stock, year, month, day;
double price;
getline(ss, name, ',');
getline(ss, author, ',');
getline(ss, publisher, ',');
ss >> pages;
ss.ignore();
ss >> year;
ss.ignore();
ss >> month;
ss.ignore();
ss >> day;
ss.ignore();
ss >> stock;
ss.ignore();
ss >> price;
Time latestTime(year, month, day);
Book b(name, author, publisher, pages, latestTime, stock, price);
books.push_back(b);
}
inFile.close();
}
```
这段代码使用 `stringstream` 对象将字符串解析成各个数据类型,相对来说更加安全可靠。
相关推荐
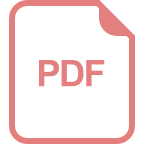
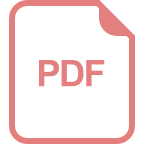












