vue3 +Django 实现所有国家地区选择
时间: 2023-11-23 20:06:53 浏览: 48
如果您想在Vue.js和Django中实现所有国家地区选择,可以考虑以下方案:
1. 在Django中使用django-countries和django-cities插件来获取国家和城市信息。您可以在Django中定义模型类来表示国家和城市信息,然后使用ORM框架来进行增删改查等操作。例如:
```python
from django_countries.fields import CountryField
from cities.models import City, Country
class UserProfile(models.Model):
# ...
country = CountryField()
city = models.ForeignKey(City, on_delete=models.PROTECT)
# ...
```
2. 在Vue.js中使用第三方的国家地区选择组件,例如vue-country-region-select、vue-area-linkage等,或者自己编写组件来实现。在组件中,您可以使用axios来向Django发送请求,获取国家和城市信息。例如:
```vue
<template>
<div>
<select v-model="selectedCountry" @change="getCities">
<option v-for="country in countries" :value="country.code">{{ country.name }}</option>
</select>
<select v-model="selectedCity">
<option v-for="city in cities" :value="city.id">{{ city.name }}</option>
</select>
</div>
</template>
<script>
import axios from 'axios';
export default {
data() {
return {
countries: [],
cities: [],
selectedCountry: '',
selectedCity: '',
};
},
methods: {
getCountries() {
axios.get('/api/countries/')
.then(response => {
this.countries = response.data;
})
.catch(error => {
console.log(error);
});
},
getCities() {
axios.get(`/api/cities/?country=${this.selectedCountry}`)
.then(response => {
this.cities = response.data;
})
.catch(error => {
console.log(error);
});
},
},
mounted() {
this.getCountries();
},
};
</script>
```
在这个示例中,我们使用了Vue.js的select元素来实现国家和城市选择。在mounted生命周期中,我们使用axios向Django发送请求,获取所有国家的信息。当用户选择一个国家时,我们使用axios再次向Django发送请求,获取该国家的城市信息。然后将这些信息绑定到select元素的选项中。
希望这个方案能对您有所帮助!
相关推荐
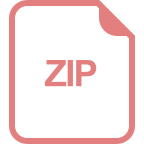
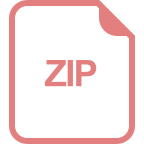














