inputs = timeseries_dataset_from_array( np.expand_dims(data_array[:-forecast_horizon], axis=-1), None, sequence_length=input_sequence_length, shuffle=False, batch_size=batch_size, )
时间: 2023-04-06 11:03:31 浏览: 60
这是一个关于机器学习的问题,我可以回答。这段代码是用来创建时间序列数据集的,它从一个数组中获取数据,然后将其转换为一个时间序列数据集,以便用于机器学习模型的训练。其中,input_sequence_length 表示输入序列的长度,batch_size 表示批次大小。
相关问题
inputs = train_data[:-1].view(1, -1, 1)
这行代码将训练数据train_data的前n-1个样本作为LSTM的输入,其中n是train_data的长度。具体来说,它首先使用切片操作train_data[:-1]将train_data中除了最后一个样本之外的所有样本提取出来,然后使用view方法将其变形为一个三维张量。第一个维度1表示batch size,第二个维度-1表示每个batch中的样本数,第三个维度1表示每个样本的特征数(在本例中为1)。这样,inputs就变成了一个形状为(1, n-1, 1)的张量,可以作为LSTM的输入。
prediction_array = np.array(inputs_data[1])什么意思
这行代码是将名为 `inputs_data` 的列表中第二个元素转换为NumPy数组,然后将其赋值给变量 `prediction_array`。假设 `inputs_data` 是一个包含多个元素的列表,那么 `inputs_data[1]` 就是该列表的第二个元素。这个元素会被转换为NumPy数组,这意味着可以使用NumPy库中的函数和方法来处理这个数组。最后,这个数组会被赋值给变量 `prediction_array`,以便在程序的后续部分中使用。
相关推荐
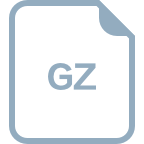
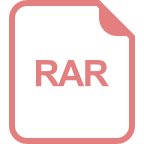
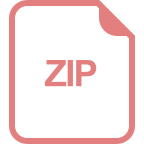












