input_shape = (None, 24) inputs = Input(shape=input_shape) # 定义TCN网络的中间层,可以设置多个卷积层和池化层 tcn_layer = TCN(nb_filters=4, kernel_size=3, dilations=[1, 2, 4])(inputs) # 定义模型,将输入层和TCN网络的中间层连接起来 model = Model(inputs=inputs, outputs=tcn_layer) # 使用predict函数获取特征,将数据分成多个batch进行处理 num_samples = x_data.shape[0] features = [] batch_size = 32 for i in range(0, num_samples, batch_size): batch = x_data[i:i + batch_size] batch = tf.expand_dims(batch, axis=0) if i + batch_size > num_samples: batch_num = num_samples - i if batch_num == 1: feature_batch = model.predict(batch.reshape(1, *input_shape), batch_size=batch_num) else: feature_batch = model.predict(batch, batch_size=batch_num) features.append(feature_batch) else: feature_batch = model.predict(batch, batch_size=batch_size) features.append(feature_batch) features = np.concatenate(features, axis=0) print(features.shape)如果想要输出长度仍为x_data[0]
时间: 2024-03-23 17:43:38 浏览: 107
如果要在输出中保留原始数据 `x_data` 的第一个维度长度,你可以在预测结束后对 `features` 进行裁剪操作,使其第一个维度的长度与 `x_data` 的第一个维度长度相同。具体来说,你可以按照以下方式修改代码:
```python
import tensorflow as tf
# 定义输入层
input_shape = (None, 24)
inputs = Input(shape=input_shape)
# 定义 TCN 网络的中间层
tcn_layer = TCN(nb_filters=4, kernel_size=3, dilations=[1, 2, 4])(inputs)
# 定义模型
model = Model(inputs=inputs, outputs=tcn_layer)
# 使用 predict 函数获取特征,将数据分成多个 batch 进行处理
num_samples = x_data.shape[0]
features = []
batch_size = 32
for i in range(0, num_samples, batch_size):
batch = x_data[i:i + batch_size]
batch = tf.expand_dims(batch, axis=0)
if i + batch_size > num_samples:
batch_num = num_samples - i
if batch_num == 1:
feature_batch = model.predict(batch.reshape(1, *input_shape), batch_size=batch_num)
else:
feature_batch = model.predict(batch, batch_size=batch_num)
features.append(feature_batch)
else:
feature_batch = model.predict(batch, batch_size=batch_size)
features.append(feature_batch)
# 将 features 沿第一个维度拼接起来
features = np.concatenate(features, axis=0)
# 对 features 进行裁剪,使其第一个维度长度与 x_data 相同
features = features[:x_data.shape[0]]
print(features.shape)
```
在上面的代码中,我们在预测结束后对 `features` 进行了裁剪操作,使其第一个维度长度与 `x_data` 相同。这样就可以保留原始数据的第一个维度长度了。
阅读全文
相关推荐
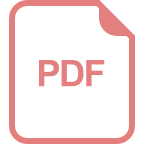
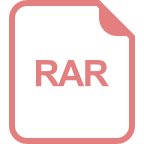
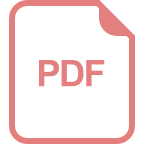
















