运用数据结构知识用c语言实现复数的四则运算
时间: 2024-09-27 15:00:47 浏览: 44
在C语言中,复数通常可以使用结构体来表示,包括实部(real)和虚部(imaginary)。以下是使用结构体以及指针来实现复数四则运算的一个简单示例:
```c
#include <stdio.h>
typedef struct {
double real;
double imag;
} Complex;
// 加法运算
Complex add(Complex a, Complex b) {
Complex result = {a.real + b.real, a.imag + b.imag};
return result;
}
// 减法运算
Complex subtract(Complex a, Complex b) {
Complex result = {a.real - b.real, a.imag - b.imag};
return result;
}
// 乘法运算
Complex multiply(Complex a, Complex b) {
double real_part = a.real * b.real - a.imag * b.imag;
double imag_part = a.real * b.imag + a.imag * b.real;
Complex result = {real_part, imag_part};
return result;
}
// 除法运算(假设分母不为零)
Complex divide(Complex a, Complex b) {
double denominator = b.real * b.real + b.imag * b.imag;
double real_part = (a.real * b.real + a.imag * b.imag) / denominator;
double imag_part = (a.imag * b.real - a.real * b.imag) / denominator;
Complex result = {real_part, imag_part};
return result;
}
int main() {
Complex num1 = {3.0, 4.0}; // 3 + 4i
Complex num2 = {1.0, 2.0}; // 1 + 2i
Complex sum = add(num1, num2);
Complex difference = subtract(num1, num2);
Complex product = multiply(num1, num2);
Complex quotient = divide(num1, num2); // 注意这里需要检查除数是否为0
printf("Sum: %.2f + %.2fi\n", sum.real, sum.imag);
printf("Difference: %.2f + %.2fi\n", difference.real, difference.imag);
printf("Product: %.2f + %.2fi\n", product.real, product.imag);
if (quotient.imag != 0) {
printf("Quotient: %.2f + %.2fi\n", quotient.real, quotient.imag);
} else {
printf("Cannot divide by zero.\n");
}
return 0;
}
```
阅读全文
相关推荐
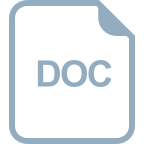
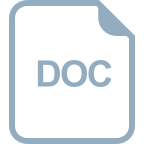
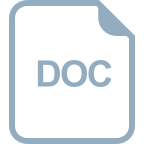

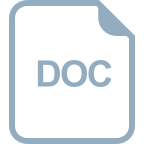
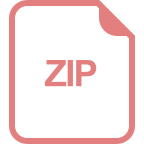
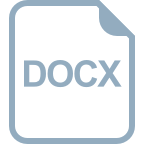
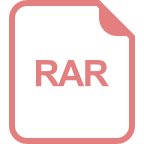
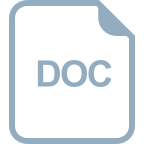
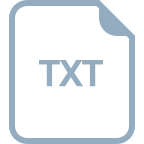
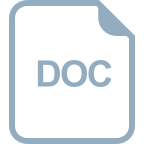


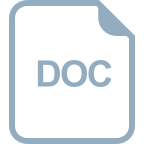

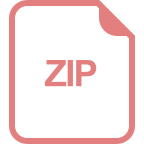