基于C语言编译实现一个对单链表进行基本操作的系统,主要包括表的创建、输入、查询、取值、插入、删除和输出操作
时间: 2023-12-20 07:04:59 浏览: 24
以下是基于C语言实现单链表的基本操作系统的代码:
```c
#include <stdio.h>
#include <stdlib.h>
//定义链表节点结构体
struct Node {
int data;
struct Node *next;
};
//创建链表
struct Node* createList(int n) {
struct Node *head, *p, *q;
int i;
head = (struct Node*)malloc(sizeof(struct Node));
p = head;
printf("请输入链表的数据:\n");
for (i = 0; i < n; i++) {
q = (struct Node*)malloc(sizeof(struct Node));
scanf("%d", &q->data);
q->next = NULL;
p->next = q;
p = q;
}
return head;
}
//插入节点
struct Node* insertNode(struct Node* head, int value, int pos) {
struct Node *p, *q;
int i;
p = head;
for (i = 0; i < pos - 1; i++) {
if (p->next == NULL) {
printf("插入位置错误!\n");
return head;
}
p = p->next;
}
q = (struct Node*)malloc(sizeof(struct Node));
q->data = value;
q->next = p->next;
p->next = q;
return head;
}
//删除节点
struct Node* deleteNode(struct Node* head, int pos) {
struct Node *p, *q;
int i;
p = head;
for (i = 0; i < pos - 1; i++) {
if (p->next == NULL) {
printf("删除位置错误!\n");
return head;
}
p = p->next;
}
q = p->next;
p->next = q->next;
free(q);
return head;
}
//查询节点
int searchNode(struct Node* head, int value) {
struct Node *p;
int pos = 0;
p = head->next;
while (p != NULL) {
pos++;
if (p->data == value) {
return pos;
}
p = p->next;
}
return -1;
}
//取值
int getValue(struct Node* head, int pos) {
struct Node *p;
int i;
p = head->next;
for (i = 0; i < pos - 1; i++) {
if (p->next == NULL) {
printf("位置错误!\n");
return -1;
}
p = p->next;
}
return p->data;
}
//输出链表
void printList(struct Node* head) {
struct Node *p;
p = head->next;
printf("链表的数据为:\n");
while (p != NULL) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
}
int main() {
int n, value, pos, choice;
struct Node *head;
printf("请输入链表的长度:");
scanf("%d", &n);
head = createList(n);
while (1) {
printf("请选择操作:\n");
printf("1、插入节点\n");
printf("2、删除节点\n");
printf("3、查询节点\n");
printf("4、取节点值\n");
printf("5、输出链表\n");
printf("6、退出\n");
scanf("%d", &choice);
switch (choice) {
case 1:
printf("请输入插入的值和位置:\n");
scanf("%d %d", &value, &pos);
head = insertNode(head, value, pos);
break;
case 2:
printf("请输入删除的位置:\n");
scanf("%d", &pos);
head = deleteNode(head, pos);
break;
case 3:
printf("请输入查询的值:\n");
scanf("%d", &value);
pos = searchNode(head, value);
if (pos != -1) {
printf("值为%d的节点的位置为%d\n", value, pos);
} else {
printf("链表中没有值为%d的节点!\n", value);
}
break;
case 4:
printf("请输入取值的位置:\n");
scanf("%d", &pos);
value = getValue(head, pos);
if (value != -1) {
printf("位置为%d的节点的值为%d\n", pos, value);
}
break;
case 5:
printList(head);
break;
case 6:
exit(0);
default:
printf("输入有误!\n");
break;
}
}
return 0;
}
```
该程序可以通过用户输入来创建一个单链表,并可进行插入、删除、查询、取值和输出操作。其中,插入、删除、查询和取值操作都需要用户输入相应的节点位置或值。程序使用switch语句实现菜单选择,用户输入对应的数字即可进行相应的操作。
相关推荐
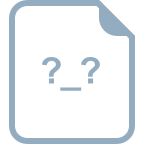
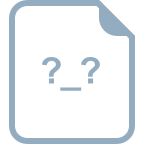














