可以给一个c++代码处理一个彩色图像的例子吗
时间: 2024-03-08 21:49:06 浏览: 70
当然可以,以下是一个基于 C++ 的生长算法分割黑色区域的示例代码:
```c++
#include <opencv2/opencv.hpp>
#include <iostream>
#include <queue>
using namespace cv;
using namespace std;
void grow(Mat& img, Point seed, int thresh, Mat& seg) {
// 定义种子点队列
queue<Point> seed_queue;
// 将种子点加入队列
seed_queue.push(seed);
// 遍历种子点队列
while (!seed_queue.empty()) {
// 取出队列中的第一个点
Point curr_seed = seed_queue.front();
seed_queue.pop();
// 将当前像素标记为黑色区域的一部分
seg.at<uchar>(curr_seed) = 255;
// 遍历相邻像素
for (int i = -1; i <= 1; i++) {
for (int j = -1; j <= 1; j++) {
// 计算相邻像素的坐标
Point next_pixel(curr_seed.x + i, curr_seed.y + j);
// 如果相邻像素未被访问过且与当前像素的差异小于阈值,则将其加入种子点队列中
if (next_pixel.x >= 0 && next_pixel.x < img.rows &&
next_pixel.y >= 0 && next_pixel.y < img.cols &&
seg.at<uchar>(next_pixel) == 0 &&
norm(img.at<Vec3b>(next_pixel) - img.at<Vec3b>(curr_seed)) < thresh) {
seed_queue.push(next_pixel);
}
}
}
}
}
int main() {
// 读入彩色图像
Mat img = imread("test.jpg");
// 转换为灰度图像
Mat gray;
cvtColor(img, gray, COLOR_BGR2GRAY);
// 设置种子点和阈值
Point seed(100, 100);
int thresh = 10;
// 分割黑色区域
Mat seg(gray.size(), CV_8UC1, Scalar(0));
grow(gray, seed, thresh, seg);
// 显示分割结果
namedWindow("Segmentation", WINDOW_NORMAL);
imshow("Segmentation", seg);
waitKey(0);
destroyAllWindows();
return 0;
}
```
在这个示例代码中,我们首先读入一张彩色图像,然后将其转换为灰度图像。接着选择一个种子点和一个阈值,调用 `grow` 函数进行生长算法分割,最后将分割结果显示出来。需要注意的是,这里的阈值是像素差异的阈值,越小则生长区域越大,越大则生长区域越小。另外,在 C++ 中需要使用 `queue` 类型来存储种子点队列。
阅读全文
相关推荐
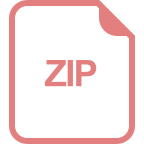
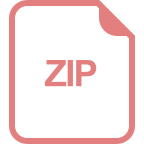
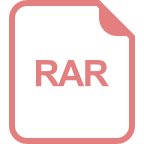
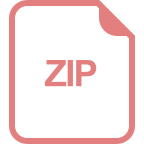
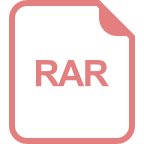
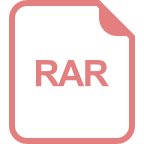
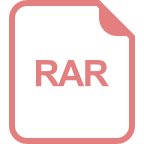
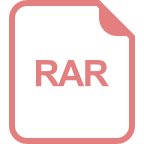
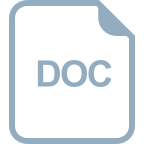
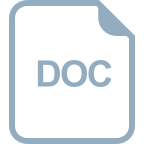
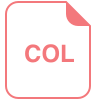
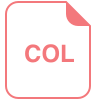
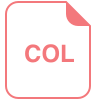



